
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
pccomms.h
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef PCCOMMS_H 00024 #define PCCOMMS_H 00025 00026 #include "sowb.h" 00027 00028 #define PCCOMMS_STATE_WAITING 0 00029 #define PCCOMMS_BASE_PACKET_READ 1 00030 #define PCCOMMS_PACKET_READ 2 00031 #define PCCOMMS_BASE_PACKET_BAD_CSUM 3 00032 00033 #define PCCOMMS_PACKET_ACCEPTED 1 00034 #define PCCOMMS_PACKET_REJECTED 2 00035 00036 typedef struct _base_packet_bin { 00037 uint16_t mode; 00038 uint16_t length; 00039 } BASE_PACKET_B; 00040 00041 #define BASE_PACKET_B_LEN sizeof(BASE_PACKET_B) 00042 00043 typedef struct _base_packet_ascii { 00044 char lead_char; 00045 char mode[4]; 00046 char length[4]; 00047 char csum; 00048 char flag; 00049 char trail_char; 00050 } BASE_PACKET_A; 00051 00052 #define BASE_PACKET_A_LEN sizeof(BASE_PACKET_A) 00053 00054 #define BASE_PACKET_WRAP if(header_packet_in>=BASE_PACKET_A_LEN)header_packet_in=0 00055 00056 void pccomms_init(void); 00057 void pccomms_process(void); 00058 00059 void Uart3_init(void); 00060 void Uart3_putc(char c); 00061 int Uart3_getc(int block); 00062 00063 void base_packet_a2b(BASE_PACKET_B *b, BASE_PACKET_A *a); 00064 void base_packet_b2a(BASE_PACKET_A *a, BASE_PACKET_B *b); 00065 00066 /* Buffer sizes MUST be a aligned 2^, for example 8, 16, 32, 64, 128, 256, 512, 1024, etc 00067 Do NOT use any other value or the buffer wrapping firmware won't work. */ 00068 #define UART3_TX_BUFFER_SIZE 512 00069 #define UART3_RX_BUFFER_SIZE 512 00070 00071 00072 #endif
Generated on Tue Jul 12 2022 18:05:35 by
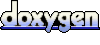