
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
mbedfilesys.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 /* Sadly stung by the Mbed libraries again. The sheer number 00024 of interrupts running on SOWB are incomp with the LocalFileSystem 00025 library which simply crashes the entire project. 00026 00027 Need to get down to the electonics shop and buy myself some flash 00028 and DIY my own solution again. Sigh... */ 00029 00030 #ifdef NEVERCOMPILETHIS 00031 00032 #include "mbed.h" 00033 #include "mbedfilesys.h" 00034 #include "user.h" 00035 #include "utils.h" 00036 #include "gpio.h" 00037 #include "debug.h" 00038 00039 LocalFileSystem local("local"); 00040 00041 inline void disable_irqs(void) { 00042 NVIC_DisableIRQ(EINT3_IRQn); 00043 NVIC_DisableIRQ(RIT_IRQn); 00044 NVIC_DisableIRQ(UART0_IRQn); 00045 NVIC_DisableIRQ(UART1_IRQn); 00046 NVIC_DisableIRQ(UART2_IRQn); 00047 NVIC_DisableIRQ(USB_IRQn); 00048 } 00049 00050 inline void enable_irqs(void) { 00051 NVIC_EnableIRQ(USB_IRQn); 00052 NVIC_EnableIRQ(EINT3_IRQn); 00053 NVIC_EnableIRQ(RIT_IRQn); 00054 NVIC_EnableIRQ(UART0_IRQn); 00055 NVIC_EnableIRQ(UART1_IRQn); 00056 NVIC_EnableIRQ(UART2_IRQn); 00057 } 00058 00059 static int get_tle_from_file(const char *filename, int index, SAT_POS_DATA *sat) { 00060 FILE *fp; 00061 char *r, line[128]; 00062 int line_count = 0, sat_count = 0; 00063 00064 fp = fopen(filename, "r"); 00065 if (!fp) { 00066 return SOWB_FILE_NOT_FOUND; 00067 } 00068 else { 00069 while (!feof(fp)) { 00070 LED1_ON; 00071 disable_irqs(); 00072 r = fgets(line, 127, fp); 00073 enable_irqs(); 00074 LED1_OFF; 00075 if(!fgets(line, 127, fp)) { 00076 fclose(fp); 00077 return SOWB_FILE_EOF_REACHED; 00078 } 00079 else { 00080 switch(line_count) { 00081 case 0: strncpy(sat->elements[0], line, 80); break; 00082 case 1: strncpy(sat->elements[1], line, 80); break; 00083 case 2: strncpy(sat->elements[2], line, 80); break; 00084 } 00085 line_count++; 00086 if (line_count == 3) { 00087 if (sat_count == index) { 00088 fclose(fp); 00089 return SOWB_FILE_OK; 00090 } 00091 line_count = 0; 00092 sat_count++; 00093 } 00094 } 00095 } 00096 } 00097 00098 fclose(fp); 00099 return SOWB_FILE_INDEX_NOT_FOUND; 00100 } 00101 00102 /* Used to hold the index across first/next calls. */ 00103 int satllite_index; 00104 00105 int get_first_tle_from_file(const char *filename, SAT_POS_DATA *sat) { 00106 satllite_index = 0; 00107 return get_tle_from_file(filename, satllite_index, sat); 00108 } 00109 00110 int get_next_tle_from_file(const char *filename, SAT_POS_DATA *sat) { 00111 satllite_index++; 00112 return get_tle_from_file(filename, satllite_index, sat); 00113 } 00114 00115 void process_tle(SAT_POS_DATA *sat) { 00116 char line[128]; 00117 00118 /* Wait until time and location are valid. */ 00119 do { 00120 observer_now(sat); 00121 user_call_process(); 00122 } 00123 while (!sat->time.is_valid || !sat->location.is_valid); 00124 00125 debug_printf(" A %d\r\n", sizeof(SAT_POS_DATA)); 00126 debug_printf(" A %s", sat->elements[0]); 00127 debug_printf(" A %s", sat->elements[1]); 00128 debug_printf(" A %s", sat->elements[2]); 00129 time_AsString(&sat->time, line); 00130 debug_printf(" A AT %s\r\n", line); 00131 sprintf(line, " A LAT %.4f\r\n", sat->location.latitude); debug_printf("%s", line); 00132 sprintf(line, " A LON %.4f\r\n", sat->location.longitude); debug_printf("%s", line); 00133 sprintf(line, " A HEI %.4f\r\n", sat->location.height); debug_printf("%s", line); 00134 00135 sat->tsince = 0; 00136 satallite_calculate(sat); 00137 debug_stringl(line, sprintf(line, " Azmith %.1f Elevation %.1f\r\n", sat->azimuth, sat->elevation)); 00138 } 00139 00140 #endif 00141 00142
Generated on Tue Jul 12 2022 18:05:35 by
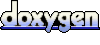