
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
main.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef MAIN_CPP 00024 #define MAIN_CPP 00025 #endif 00026 00027 #include "sowb.h" 00028 #include "gpioirq.h" 00029 #include "gpio.h" 00030 #include "rit.h" 00031 #include "usbeh.h" 00032 #include "usbeh_endpoint.h" 00033 #include "usbeh_device.h" 00034 #include "usbeh_controller.h" 00035 #include "usbeh_api.h" 00036 #include "xbox360gamepad.h" 00037 #include "th_xbox360gamepad.h" 00038 #include "gps.h" 00039 #include "MAX7456.h" 00040 #include "osd.h" 00041 #include "nexstar.h" 00042 #include "utils.h" 00043 #include "user.h" 00044 #include "dma.h" 00045 #include "flash.h" 00046 #include "sdcard.h" 00047 #include "config.h" 00048 #include "ff.h" 00049 #include "sgp4sdp4.h" 00050 #include "satapi.h" 00051 #include "star.h" 00052 00053 #include "main.h" 00054 #include "debug.h" 00055 #include "predict_th.h" 00056 00057 int test_flash_page; 00058 00059 /* Create an array of _process function pointers 00060 to call while user_io is waiting. */ 00061 typedef void (PROCESS_FUNC)(); 00062 00063 PROCESS_FUNC *process_callbacks[] = { 00064 usbeh_api_process, 00065 xbox360gamepad_process, 00066 gps_process, 00067 gpioirq_process, 00068 nexstar_process, 00069 sdcard_process, 00070 config_process, 00071 NULL 00072 }; 00073 00074 int main_test_flag; 00075 00076 void _main_test_callback(int index) { 00077 main_test_flag = 0; 00078 } 00079 00080 00081 00082 int main() { 00083 int counter = 0; 00084 char test_buffer[256]; 00085 DIR fDir; 00086 FILINFO fInfo; 00087 int f_return; 00088 GPS_LOCATION_RAW location; 00089 00090 /* Carry out module start-up _init() functions. 00091 Note, the order is important, do not change. */ 00092 debug_init(); 00093 gpio_init(); 00094 rit_init(); 00095 xbox360gamepad_init(); 00096 usbeh_api_init(); 00097 MAX7456_init(); 00098 osd_init(); 00099 gps_init(); 00100 gpioirq_init(); 00101 00102 /* We use raw MAX7456 calls to display the splash screen because 00103 at this point not all interrupts are active and the OSD system 00104 will not yet be fully operational even though we've _init() it. */ 00105 MAX7456_cursor(0, 5); MAX7456_string((unsigned char *)" Satellite Observers "); 00106 MAX7456_cursor(0, 6); MAX7456_string((unsigned char *)" Workbench V0.1 "); 00107 MAX7456_cursor(0, 7); MAX7456_string((unsigned char *)" (c) Copyright 2010 "); 00108 MAX7456_cursor(0, 8); MAX7456_string((unsigned char *)" Stellar Technologies Ltd"); 00109 if (LPC_WDT->WDMOD & 0x4) { MAX7456_cursor(0, 14); MAX7456_string((unsigned char *)" WDT Error detected"); } 00110 user_wait_ms_blocking(2000); /* Simple splash screen delay. */ 00111 MAX7456_cursor(0, 11); MAX7456_string((unsigned char *)" Press A to continue"); 00112 while (user_get_button(false) != BUTT_A_PRESS) ; 00113 while (user_get_button(false) != BUTT_A_RELEASE) ; 00114 00115 /* Complete the module _init() stage. */ 00116 nexstar_init(); 00117 DMA_init(); 00118 flash_init(); 00119 sdcard_init(); 00120 config_init(); 00121 th_xbox360gamepad_init(); 00122 00123 if (!_nexstar_is_aligned()) { 00124 debug_printf("Nexstar not aligned, forcing user to align.\r\n"); 00125 nexstar_force_align(); 00126 } 00127 00128 MAX7456_cursor(0, 11); MAX7456_string((unsigned char *)" Waiting for GPS...."); 00129 do { 00130 gps_get_location_raw(&location); 00131 WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00132 } while (location.is_valid == '0'); 00133 osd_clear(); osd_set_mode_l01(L01_MODE_A); 00134 00135 /* Tell the Nexstar the real time and place. */ 00136 _nexstar_set_time(NULL); 00137 _nexstar_set_location(NULL); 00138 00139 /* Init the watchdog and then go into the main loop. */ 00140 LPC_SC->PCLKSEL0 |= 0x3; 00141 LPC_WDT->WDCLKSEL = 1; 00142 LPC_WDT->WDTC = 6000000; 00143 LPC_WDT->WDMOD = 3; 00144 KICK_WATCHDOG; 00145 00146 while(1) { 00147 00148 char c = user_get_button(false); 00149 switch (c) { 00150 case BUTT_START_PRESS: 00151 if (!sdcard_is_mounted()) { 00152 osd_string_xy(1, 14, "No SD card inserted"); 00153 } 00154 break; 00155 00156 case BUTT_XBOX_PRESS: 00157 SAT_POS_DATA q; 00158 satapi_aos(&q, true); 00159 break; 00160 00161 case BUTT_LS_PRESS: 00162 osd_l01_next_mode(); 00163 break; 00164 case BUTT_RS_PRESS: 00165 osd_crosshair_toggle(); 00166 break; 00167 case BUTT_B_PRESS: 00168 //_nexstar_goto_azm_fast(0x238F); 00169 //_nexstar_goto(0x238F, 0x238F); 00170 _nexstar_goto(0x0, 0x0); 00171 break; 00172 case BUTT_X_PRESS: 00173 /* 00174 _nexstar_set_elevation_rate_auto(1.0); 00175 main_test_flag = 1; 00176 rit_timer_set_counter(MAIN_TEST_CB, 5000); 00177 P22_ASSERT; 00178 while (main_test_flag == 1) { 00179 user_call_process(); 00180 } 00181 _nexstar_set_elevation_rate_auto(0); 00182 P22_DEASSERT; 00183 */ 00184 00185 for (int i = 0; i < 256; i++) { 00186 test_buffer[i] = 255 - i; 00187 } 00188 debug_printf("Test buffer before:-\r\n"); 00189 printBuffer(test_buffer, 256); 00190 flash_page_write(1, test_buffer); 00191 LED1_ON; 00192 while(flash_write_in_progress()); 00193 LED1_OFF; 00194 flash_read_page(1, test_buffer, true); 00195 debug_printf("Test buffer after:-\r\n"); 00196 printBuffer(test_buffer, 256); 00197 break; 00198 00199 case BUTT_Y_PRESS: 00200 memset(test_buffer, 0xAA, 256); 00201 flash_read_page(0, test_buffer, true); 00202 debug_printf("Page 0\r\n"); 00203 printBuffer(test_buffer, 256); 00204 memset(test_buffer, 0xAA, 256); 00205 flash_read_page(1, test_buffer, true); 00206 debug_printf("Page 1\r\n"); 00207 printBuffer(test_buffer, 256); 00208 memset(test_buffer, 0xAA, 256); 00209 flash_read_page(2, test_buffer, true); 00210 debug_printf("Page 2\r\n"); 00211 printBuffer(test_buffer, 256); 00212 memset(test_buffer, 0xAA, 256); 00213 flash_read_page(3, test_buffer, true); 00214 debug_printf("Page 3\r\n"); 00215 printBuffer(test_buffer, 256); 00216 break; 00217 case BUTT_DPAD_DOWN_PRESS: 00218 flash_erase_sector(0); 00219 break; 00220 case BUTT_DPAD_UP_PRESS: 00221 flash_erase_bulk(); 00222 break; 00223 case BUTT_DPAD_LEFT_PRESS: 00224 { 00225 AltAz y; 00226 RaDec x; 00227 GPS_LOCATION_AVERAGE loc; 00228 GPS_TIME t; 00229 memset(&t, 0, sizeof(GPS_TIME)); 00230 t.year = 2010; t.month = 10; t.day = 7; t.hour = 21; t.minute = 15; t.second = 21; 00231 00232 double jd = gps_julian_date(&t); 00233 sprintf(test_buffer, "\n\n\rJD = %f\r\n", jd); 00234 debug_printf(test_buffer); 00235 00236 gps_get_location_average(&loc); 00237 double siderealDegrees = gps_siderealDegrees_by_time(&t); 00238 sprintf(test_buffer, "SR by time = %f\r\n", siderealDegrees); 00239 debug_printf(test_buffer); 00240 00241 x.dec = 28.026111; 00242 x.ra = 116.32875; 00243 00244 sprintf(test_buffer, "Staring with Dec = %f, RA = %f\r\n", x.dec, x.ra); 00245 debug_printf(test_buffer); 00246 00247 radec2altaz(siderealDegrees, &loc, &x, &y); 00248 sprintf(test_buffer, "Alt = %f, Azm = %f\r\n", y.alt, y.azm); 00249 debug_printf(test_buffer); 00250 00251 altaz2radec(siderealDegrees, &loc, &y, &x); 00252 sprintf(test_buffer, "Dec = %f, RA = %f\r\n", x.dec, x.ra); 00253 debug_printf(test_buffer); 00254 00255 // Lets look for the brightest star near Sirius. 00256 // It should return HR2491, Sirius it's self. 00257 // RA 6 45 8.9 101.2606833 00258 // Dec -16 42, 58 -16.716111 00259 x.ra = 101.2606833; x.dec = -16.716111; 00260 char test_buffer2[32]; 00261 basicStarData star, *p; 00262 p = star_closest(&x, &star); 00263 if (!p) { 00264 debug_printf("No star found\r\n"); 00265 } 00266 else { 00267 sprintf(test_buffer, "HR%d %f %f %f\r\n", star.hr, star.ra, star.dec, star.mag); 00268 debug_printf(test_buffer); 00269 } 00270 00271 00272 } 00273 00274 00275 break; 00276 } 00277 00278 //sgp4sdp4_th_init(); 00279 00280 /* 00281 for(int i = 0; process_callbacks[i] != NULL; i++) { 00282 (process_callbacks[i])(); 00283 } 00284 00285 th_xbox360gamepad(); 00286 */ 00287 } 00288 } 00289
Generated on Tue Jul 12 2022 18:05:35 by
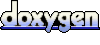