
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
gps.h
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef GPS_H 00024 #define GPS_H 00025 00026 #define GPS_LAT_STR 0 00027 #define GPS_LON_STR 1 00028 00029 #define GPS_HISTORY_SIZE 60 00030 00031 typedef struct _gps_time { 00032 int year; 00033 char month; 00034 char day; 00035 char hour; 00036 char minute; 00037 char second; 00038 char tenth; 00039 char hundreth; 00040 char is_valid; 00041 char prev_valid; 00042 } GPS_TIME; 00043 00044 typedef struct _gps_location_raw { 00045 char north_south; 00046 char east_west; 00047 char lat[16]; 00048 char lon[16]; 00049 char alt[16]; 00050 char sats[8]; 00051 char is_valid; 00052 uint32_t updated; 00053 } GPS_LOCATION_RAW; 00054 00055 typedef struct _gps_location_average { 00056 char north_south; 00057 double latitude; 00058 char east_west; 00059 double longitude; 00060 double height; 00061 char *sats; 00062 char is_valid; 00063 } GPS_LOCATION_AVERAGE; 00064 00065 /* GPS module API function prototypes. */ 00066 void gps_init(void); 00067 void gps_process(void); 00068 double gps_convert_coord(char *s, int type); 00069 double gps_julian_day_number(GPS_TIME *t); 00070 double gps_julian_date(GPS_TIME *t); 00071 double gps_siderealDegrees_by_jd(double jd); 00072 double gps_siderealDegrees_by_time(GPS_TIME *t); 00073 double gps_siderealHA_by_jd(double jd); 00074 double gps_siderealHA_by_time(GPS_TIME *t); 00075 GPS_TIME *gps_get_time(GPS_TIME *q); 00076 GPS_LOCATION_RAW *gps_get_location_raw(GPS_LOCATION_RAW *q); 00077 GPS_LOCATION_AVERAGE *gps_get_location_average(GPS_LOCATION_AVERAGE *q); 00078 00079 /* Used by other modules to make callbacks. */ 00080 void gps_pps_fall(void); /* gpioirq.c needs this to know what to callback to. */ 00081 00082 #define GPS_BUFFER_SIZE 128 00083 00084 /* Used to test the IIR register. Common across UARTs. */ 00085 #define UART_ISSET_THRE 0x0002 00086 #define UART_ISSET_RDA 0x0004 00087 #define UART_ISSET_CTI 0x000C 00088 #define UART_ISSET_RLS 0x0006 00089 #define UART_ISSET_FIFOLVL_RXFULL 0x0000000F 00090 #define UART_ISSET_FIFOLVL_TXFULL 0x00000F00 00091 00092 #define UART_RX_INTERRUPT iir & UART_ISSET_RDA 00093 00094 00095 /* UART1 register configuration values. */ 00096 #define UART1_SET_LCR 0x00000003 00097 #define UART1_SET_LCR_DLAB 0x00000083 00098 #define UART1_SET_DLLSB 0x71 00099 #define UART1_SET_DLMSB 0x02 00100 #define UART1_SET_FCR 0x01 00101 #define UART1_SET_FCR_CLEAR 0x07 00102 #define UART1_SET_IER 0x07 00103 00104 /* Macros. */ 00105 #define UART1_FIFO_NOT_EMPTY LPC_UART1->LSR & 0x1 00106 #define UART1_GETC (char)LPC_UART1->RBR 00107 00108 #endif 00109
Generated on Tue Jul 12 2022 18:05:35 by
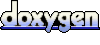