
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
gpio.h
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef GPIO_H 00024 #define GPIO_H 00025 00026 /* Switch on our macros to use the 4 LEDs on the Mbed. */ 00027 #define MBED_LEDS 00028 00029 /* Used by the MAX7456 module. 00030 The MAX7456 !cs signal is connected to Mbed pin 8 00031 which is internally connected to Port0.6 */ 00032 #define SET_P0_06 (LPC_GPIO0->FIOSET = (1UL << 6)) 00033 #define CLR_P0_06 (LPC_GPIO0->FIOCLR = (1UL << 6)) 00034 #define VAL_P0_06 (LPC_GPIO0->FIOPIN & (1UL << 6)) 00035 #define MAX7456_CS_ASSERT CLR_P0_06 00036 #define MAX7456_CS_DEASSERT SET_P0_06 00037 #define MAX7456_CS_VALUE VAL_P0_06 00038 #define MAX7456_CS_TOGGLE MAX7456_CS_VALUE ? MAX7456_CS_DEASSERT : MAX7456_CS_ASSERT 00039 00040 /* Used by the MAX7456 module. 00041 The MAX7456 !rst signal is connected to Mbed pin 20 00042 which is internally connected to Port1.31 */ 00043 #define SET_P1_31 (LPC_GPIO1->FIOSET = (1UL << 31)) 00044 #define CLR_P1_31 (LPC_GPIO1->FIOCLR = (1UL << 31)) 00045 #define VAL_P1_31 (LPC_GPIO1->FIOPIN & (1UL << 31)) 00046 #define MAX7456_RST_ASSERT CLR_P1_31 00047 #define MAX7456_RST_DEASSERT SET_P1_31 00048 #define MAX7456_RST_VALUE VAL_P1_31 00049 #define MAX7456_RST_TOGGLE MAX7456_RST_VALUE ? MAX7456_RST_DEASSERT : MAX7456_RST_ASSERT 00050 #define SSP0_CS_ASSERT CLR_P1_31 00051 #define SSP0_CS_DEASSERT SET_P1_31 00052 #define SSP0_CS_VALUE VAL_P1_31 00053 #define SSP0_CS_TOGGLE MAX7456_RST_VALUE ? MAX7456_RST_DEASSERT : MAX7456_RST_ASSERT 00054 00055 /* Used by the flash module. 00056 The flash !cs signal is connected to Mbed pin 14 00057 which is internally connected to Port0.16 */ 00058 #define SET_P0_16 (LPC_GPIO0->FIOSET = (1UL << 16)) 00059 #define CLR_P0_16 (LPC_GPIO0->FIOCLR = (1UL << 16)) 00060 #define VAL_P0_16 (LPC_GPIO0->FIOPIN & (1UL << 16)) 00061 #define FLASH_CS_ASSERT CLR_P0_16 00062 #define FLASH_CS_DEASSERT SET_P0_16 00063 #define FLASH_CS_VALUE VAL_P0_16 00064 #define FLASH_CS_TOGGLE FLASH_CS_VALUE ? FLASH_CS_DEASSERT : FLASH_CS_ASSERT 00065 00066 /* Used by the 25AA02E48 module. 00067 The device !cs signal is connected to Mbed pin 16 00068 which is internally connected to Port0.24 */ 00069 #define SET_P0_24 (LPC_GPIO0->FIOSET = (1UL << 24)) 00070 #define CLR_P0_24 (LPC_GPIO0->FIOCLR = (1UL << 24)) 00071 #define VAL_P0_24 (LPC_GPIO0->FIOPIN & (1UL << 24)) 00072 #define AA02E48_CS_ASSERT CLR_P0_24 00073 #define AA02E48_CS_DEASSERT SET_P0_24 00074 #define AA02E48_CS_VALUE VAL_P0_24 00075 #define AA02E48_CS_TOGGLE AA02E48_CS_VALUE ? AA02E48_CS_DEASSERT : AA02E48_CS_ASSERT 00076 00077 /* Used by the SD card. 00078 The device !cs signal is connected to Mbed pin 19 00079 which is internally connected to Port1.30 */ 00080 #define SET_P1_30 (LPC_GPIO1->FIOSET = (1UL << 30)) 00081 #define CLR_P1_30 (LPC_GPIO1->FIOCLR = (1UL << 30)) 00082 #define VAL_P1_30 (LPC_GPIO1->FIOPIN & (1UL << 30)) 00083 #define SDCARD_CS_ASSERT CLR_P1_30 00084 #define SDCARD_CS_DEASSERT SET_P1_30 00085 #define SDCARD_CS_VALUE VAL_P1_30 00086 #define SDCARD_CS_TOGGLE SDCARD_CS_VALUE ? SDCARD_CS_DEASSERT : SDCARD_CS_ASSERT 00087 00088 /* Used for reading the SD Card detect pin. */ 00089 #define SDCARD_DETECT (LPC_GPIO0->FIOPIN & (1UL << 25)) 00090 00091 /* For debugging. 00092 Mbed pin 21 which is internally connected to Port2.5 */ 00093 #define SET_P2_05 (LPC_GPIO2->FIOSET = (1UL << 5)) 00094 #define CLR_P2_05 (LPC_GPIO2->FIOCLR = (1UL << 5)) 00095 #define VAL_P2_05 (LPC_GPIO2->FIOPIN & (1UL << 5)) 00096 #define P21_ASSERT SET_P2_05 00097 #define P21_DEASSERT CLR_P2_05 00098 #define P21_VALUE VAL_P2_05 00099 #define P21_TOGGLE P21_VALUE ? P21_DEASSERT : P21_ASSERT 00100 00101 /* For debugging. 00102 Mbed pin 22 which is internally connected to Port2.4 */ 00103 #define SET_P2_04 (LPC_GPIO2->FIOSET = (1UL << 4)) 00104 #define CLR_P2_04 (LPC_GPIO2->FIOCLR = (1UL << 4)) 00105 #define VAL_P2_04 (LPC_GPIO2->FIOPIN & (1UL << 4)) 00106 #define P22_ASSERT SET_P2_04 00107 #define P22_DEASSERT CLR_P2_04 00108 #define P22_VALUE VAL_P2_04 00109 #define P22_TOGGLE P22_VALUE ? P22_DEASSERT : P22_ASSERT 00110 00111 #ifdef MBED_LEDS 00112 #define LED1_ON (LPC_GPIO1->FIOSET = (1UL << 18)) 00113 #define LED1_OFF (LPC_GPIO1->FIOCLR = (1UL << 18)) 00114 #define LED1_IS_ON (LPC_GPIO1->FIOPIN & (1UL << 18)) 00115 #define LED1_TOGGLE LED1_IS_ON ? LED1_OFF : LED1_ON 00116 #define LED2_ON (LPC_GPIO1->FIOSET = (1UL << 20)) 00117 #define LED2_OFF (LPC_GPIO1->FIOCLR = (1UL << 20)) 00118 #define LED2_IS_ON (LPC_GPIO1->FIOPIN & (1UL << 20)) 00119 #define LED2_TOGGLE LED2_IS_ON ? LED2_OFF : LED2_ON 00120 #define LED3_ON (LPC_GPIO1->FIOSET = (1UL << 21)) 00121 #define LED3_OFF (LPC_GPIO1->FIOCLR = (1UL << 21)) 00122 #define LED3_IS_ON (LPC_GPIO1->FIOPIN & (1UL << 21)) 00123 #define LED3_TOGGLE LED3_IS_ON ? LED3_OFF : LED3_ON 00124 #define LED4_ON (LPC_GPIO1->FIOSET = (1UL << 23)) 00125 #define LED4_OFF (LPC_GPIO1->FIOCLR = (1UL << 23)) 00126 #define LED4_IS_ON (LPC_GPIO1->FIOPIN & (1UL << 23)) 00127 #define LED4_TOGGLE LED4_IS_ON ? LED4_OFF : LED4_ON 00128 #endif 00129 00130 /* Function prototypes. */ 00131 void gpio_init(void); 00132 void gpio_process(void); 00133 00134 #endif 00135
Generated on Tue Jul 12 2022 18:05:35 by
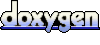