
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
gpio.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "sowb.h" 00024 #include "debug.h" 00025 #include "gpio.h" 00026 00027 00028 /** gpio_init 00029 */ 00030 void gpio_init(void) { 00031 00032 DEBUG_INIT_START; 00033 00034 /* The following code could be condensed into a single set of 00035 and/or statements. However, for code clarity they are laid 00036 out on a "use case" way to see each pin being defined. Since 00037 this is "init" code and only called once at start-up, the 00038 extra overhead isn't worth the effort over cleaner to read 00039 code. */ 00040 00041 /* The MAX7456 module uses p0.23 (p15) for it's chip select 00042 output. Define it's setup here and use the macros in gpio.h 00043 to assert, deassert or read the pin. */ 00044 //LPC_PINCON->PINSEL1 &= ~(3UL << 14); /* Function GPIO. */ 00045 //LPC_GPIO0->FIODIR |= (1UL << 23); /* P0.23 as output. */ 00046 00047 /* The MAX7456 module uses p0.16 (p14) for it's chip select 00048 output. Define it's setup here and use the macros in gpio.h 00049 to assert, deassert or read the pin. */ 00050 //LPC_PINCON->PINSEL1 &= ~(3UL << 14); /* Function GPIO. */ 00051 //LPC_GPIO0->FIODIR |= (1UL << 16); /* P0.23 as output. */ 00052 00053 /* The MAX7456 module uses p0.6 (p8) for it's chip select 00054 output. Define it's setup here and use the macros in gpio.h 00055 to assert, deassert or read the pin. */ 00056 LPC_PINCON->PINSEL0 &= ~(3UL << 12); /* Function GPIO. */ 00057 LPC_GPIO0->FIODIR |= (1UL << 6); /* P0.6 as output. */ 00058 00059 00060 /* The MAX7456 module uses p1.31 (p20) for it's reset output. 00061 Define it's setup here and use the macros in gpio.h to assert, 00062 deassert or read the pin. */ 00063 LPC_PINCON->PINSEL3 &= ~(3UL << 30); /* Function GPIO. */ 00064 LPC_GPIO1->FIODIR |= (1UL << 31); /* P1.31 as output. */ 00065 00066 /* We use p0.25 (p17) for the SD Card detect. */ 00067 LPC_PINCON->PINSEL1 &= ~(3UL << 18); /* Function GPIO. */ 00068 LPC_GPIO0->FIODIR &= ~(1UL << 25); /* P0.25 as Input. */ 00069 00070 /* We use p0.16 (p14) for the Flash device SSP0 CS signal. */ 00071 LPC_PINCON->PINSEL1 &= ~(3UL << 14); /* Function GPIO. */ 00072 LPC_GPIO0->FIODIR |= (1UL << 16); /* P0.23 as output. */ 00073 00074 /* We use p0.24 (p16) for the 25AA02E48 device SSP0 CS signal. */ 00075 LPC_PINCON->PINSEL1 &= ~(3UL << 14); /* Function GPIO. */ 00076 LPC_GPIO0->FIODIR |= (1UL << 24); /* P0.24 as output. */ 00077 00078 /* We use p1.30 (p19) for the MicroSD card device SSP0 CS signal. */ 00079 LPC_PINCON->PINSEL3 &= ~(3UL << 28); /* Function GPIO. */ 00080 LPC_GPIO1->FIODIR |= (1UL << 30); /* P1.30 as output. */ 00081 00082 /* We use p2.5 (p21) for debugging. */ 00083 LPC_PINCON->PINSEL4 &= ~(3UL << 10); /* Function GPIO. */ 00084 LPC_GPIO2->FIODIR |= (1UL << 5); /* P2.5 as output. */ 00085 00086 /* We use p2.4 (p22) for debugging. */ 00087 LPC_PINCON->PINSEL4 &= ~(3UL << 8); /* Function GPIO. */ 00088 LPC_GPIO2->FIODIR |= (1UL << 4); /* P2.4 as output. */ 00089 00090 00091 #ifdef MBED_LEDS 00092 /* The MBED has four useful little blue LEDs that can be used. 00093 Mbed examples use the DigitalOut led1(LED1) style. Mimic that 00094 using our system here. Here however, I will use shorthand ;) 00095 LED1 LED2 LED3 LED4 */ 00096 LPC_PINCON->PINSEL3 &= ( ~(3UL << 4) & ~(3UL << 8) & ~(3UL << 10) & ~(3UL << 14) ); 00097 LPC_GPIO1->FIODIR |= ( (1UL << 18) | (1UL << 20) | (1UL << 21) | (1UL << 23) ); 00098 #endif 00099 00100 00101 SSP0_CS_DEASSERT; 00102 FLASH_CS_DEASSERT; 00103 SDCARD_CS_DEASSERT; 00104 AA02E48_CS_DEASSERT; 00105 MAX7456_CS_DEASSERT; 00106 00107 DEBUG_INIT_END; 00108 } 00109 00110 /** gpio_process 00111 */ 00112 void gpio_process(void) { 00113 /* Does nothing, no house keeping required. */ 00114 } 00115
Generated on Tue Jul 12 2022 18:05:35 by
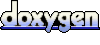