
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
flash_erase.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "sowb.h" 00024 #include "flash.h" 00025 #include "ssp0.h" 00026 #include "dma.h" 00027 #include "gpio.h" 00028 #include "rit.h" 00029 #include "user.h" 00030 #include "utils.h" 00031 #include "debug.h" 00032 00033 /* Flags to show what state we are in. */ 00034 bool sector_erase_in_progress = false; 00035 extern bool page_write_in_progress; 00036 00037 bool flash_sector_erase_in_progress(void) { 00038 return sector_erase_in_progress; 00039 } 00040 00041 /** flash_erase_sector 00042 */ 00043 int flash_erase_sector(int sector) { 00044 00045 /* If a sector erase is in progress already 00046 we return zero rather than wait (block) 00047 because an erase can take so long to complete 00048 we don't want to hang around waiting. Let the 00049 caller reschedule it sometime later. */ 00050 if (sector_erase_in_progress) { 00051 return 0; 00052 } 00053 00054 /* Request use of SSP0. */ 00055 while(!SSP0_request()) WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00056 00057 sector_erase_in_progress = true; 00058 00059 FLASH_CS_ASSERT; 00060 FLASH_SHORT_COMMAND(FLASH_WREN); 00061 FLASH_CS_DEASSERT; 00062 00063 SSP0_FLUSH_RX_FIFO; 00064 00065 /* Wait until the flash device has the WEL bit on. */ 00066 while ((LPC_SSP0->DR & 0x2) == 0) { 00067 FLASH_CS_ASSERT; 00068 FLASH_SHORT_COMMAND(FLASH_RDSR); 00069 SSP0_FLUSH_RX_FIFO; 00070 SSP0_WRITE_BYTE(0); 00071 while (SSP0_IS_BUSY); 00072 FLASH_CS_DEASSERT; 00073 } 00074 00075 SSP0_FLUSH_RX_FIFO; 00076 00077 FLASH_CS_ASSERT; 00078 FLASH_LONG_COMMAND(FLASH_SE, sector); 00079 FLASH_CS_DEASSERT; 00080 00081 /* Note, a sector erase takes much longer than 00082 a page write (typical 600ms by the datasheet) 00083 so there's no point making the first timeout 00084 very short and producing a lot of uneeded 00085 interrupts. So we set the first timeout to 00086 be 600 and then it'll switch to a much shorter 00087 time in the ISR. */ 00088 rit_timer_set_counter(FLASH_WRITE_CB, 600); 00089 return 1; 00090 } 00091 00092 /** flash_erase_bulk 00093 */ 00094 int flash_erase_bulk(void) { 00095 00096 /* If a sector erase is in progress already 00097 we return zero rather than wait (block) 00098 because an erase can take so long to complete 00099 we don't want to hang around waiting. Let the 00100 caller reschedule it sometime later. */ 00101 00102 if (sector_erase_in_progress || page_write_in_progress) { 00103 return 0; 00104 } 00105 00106 /* Request use of SSP0. */ 00107 while(!SSP0_request()) WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00108 00109 sector_erase_in_progress = true; 00110 00111 FLASH_CS_ASSERT; 00112 FLASH_SHORT_COMMAND(FLASH_WREN); 00113 FLASH_CS_DEASSERT; 00114 00115 SSP0_FLUSH_RX_FIFO; 00116 00117 /* Wait until the flash device has the WEL bit on. */ 00118 while ((LPC_SSP0->DR & 0x2) == 0) { 00119 FLASH_CS_ASSERT; 00120 FLASH_SHORT_COMMAND(FLASH_RDSR); 00121 SSP0_FLUSH_RX_FIFO; 00122 SSP0_WRITE_BYTE(0); 00123 while (SSP0_IS_BUSY); 00124 FLASH_CS_DEASSERT; 00125 } 00126 00127 FLASH_CS_ASSERT; 00128 FLASH_SHORT_COMMAND(FLASH_BE); 00129 FLASH_CS_DEASSERT; 00130 00131 /* Note, a bulk erase takes much longer than 00132 a page write (typical 8s by the datasheet) 00133 so there's no point making the first timeout 00134 very short and producing a lot of uneeded 00135 interrupts. So we set the first timeout to 00136 be 8000 and then it'll switch to a much shorter 00137 time in the ISR. */ 00138 rit_timer_set_counter(FLASH_WRITE_CB, 8000); 00139 return 1; 00140 } 00141
Generated on Tue Jul 12 2022 18:05:35 by
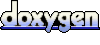