
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
flash.h
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef FLASH_H 00024 #define FLASH_H 00025 00026 #define FLASH_PAGE_SIZE 256 00027 00028 #define FLASH_WIP_TEST_TIME 2 00029 00030 #define FLASH_WREN 0x06 00031 #define FLASH_WRDI 0x04 00032 #define FLASH_RDID 0x9F 00033 #define FLASH_RDSR 0x05 00034 #define FLASH_WRSR 0x01 00035 #define FLASH_READ 0x03 00036 #define FLASH_FAST_READ 0x0B 00037 #define FLASH_PP 0x02 00038 #define FLASH_SE 0xD8 00039 #define FLASH_BE 0xC7 00040 00041 #define DMA_CHANNEL_ENABLE 1 00042 //#define DMA_CHANNEL_SRC_PERIPHERAL_SSP1_RX (3UL << 1) 00043 //#define DMA_CHANNEL_SRC_PERIPHERAL_SSP1_TX (2UL << 1) 00044 //#define DMA_CHANNEL_DST_PERIPHERAL_SSP1_RX (3UL << 6) 00045 //#define DMA_CHANNEL_DST_PERIPHERAL_SSP1_TX (2UL << 6) 00046 #define DMA_CHANNEL_SRC_PERIPHERAL_SSP0_RX (1UL << 1) 00047 #define DMA_CHANNEL_SRC_PERIPHERAL_SSP0_TX (0UL << 1) 00048 #define DMA_CHANNEL_DST_PERIPHERAL_SSP0_RX (1UL << 6) 00049 #define DMA_CHANNEL_DST_PERIPHERAL_SSP0_TX (0UL << 6) 00050 #define DMA_CHANNEL_SRC_INC (1UL << 26) 00051 #define DMA_CHANNEL_DST_INC (1UL << 27) 00052 #define DMA_CHANNEL_TCIE (1UL << 31) 00053 #define DMA_TRANSFER_TYPE_M2M (0UL << 11) 00054 #define DMA_TRANSFER_TYPE_M2P (1UL << 11) 00055 #define DMA_TRANSFER_TYPE_P2M (2UL << 11) 00056 #define DMA_TRANSFER_TYPE_P2P (3UL << 11) 00057 #define DMA_MASK_IE (1UL << 14) 00058 #define DMA_MASK_ITC (1UL << 15) 00059 #define DMA_LOCK (1UL << 16) 00060 #define DMA_ACTIVE (1UL << 17) 00061 #define DMA_HALT (1UL << 18) 00062 00063 #define FLASH_SHORT_COMMAND(x) \ 00064 SSP0_WRITE_BYTE(x); \ 00065 while(SSP0_IS_BUSY); \ 00066 SSP0_FLUSH_RX_FIFO; 00067 00068 #define FLASH_LONG_COMMAND(x,y) \ 00069 SSP0_WRITE_BYTE(x); \ 00070 SSP0_WRITE_BYTE((y >> 8) & (0xFF)); \ 00071 SSP0_WRITE_BYTE(y & 0xFF); \ 00072 SSP0_WRITE_BYTE(0); \ 00073 while(SSP0_IS_BUSY); \ 00074 SSP0_FLUSH_RX_FIFO; 00075 00076 #define FLASH_WAIT_WHILE_WIP \ 00077 SSP0_CS_ASSERT; \ 00078 FLASH_SHORT_COMMAND(FLASH_RDSR); \ 00079 SSP0_FLUSH_RX_FIFO; \ 00080 do { \ 00081 SSP0_WRITE_BYTE(0); \ 00082 } \ 00083 while (LPC_SSP0->DR & 0x1); \ 00084 SSP0_CS_DEASSERT; 00085 00086 00087 /* Defined in flash.c */ 00088 void flash_init(void); 00089 void flash_process(void); 00090 char flash_getc(bool peek); 00091 void flash_seek(unsigned int addr); 00092 00093 /* Defined in flash_read.c */ 00094 bool flash_read_in_progress(void); 00095 void flash_read_page(unsigned int page_address, char *buffer, bool block); 00096 00097 /* Defined in flash_write.c */ 00098 int flash_erase_sector(int sector); 00099 bool flash_write_in_progress(void); 00100 bool flash_sector_erase_in_progress(void); 00101 int flash_page_write(int page, char *buffer); 00102 00103 /* Defined in flash_erase.c */ 00104 bool flash_sector_erase_in_progress(void); 00105 int flash_erase_sector(int sector); 00106 int flash_erase_bulk(void); 00107 00108 /* Defined in 25AA02E44.c */ 00109 void _25AA02E48_init(void); 00110 void _25AA02E48_mac_addr(char *); 00111 void _25AA02E48_mac_addr_printable(char *, char); 00112 00113 #endif
Generated on Tue Jul 12 2022 18:05:35 by
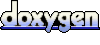