
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
flash.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "sowb.h" 00024 #include "debug.h" 00025 #include "ssp0.h" 00026 #include "gpio.h" 00027 #include "flash.h" 00028 #include "user.h" 00029 00030 extern bool sector_erase_in_progress; 00031 extern bool page_write_in_progress; 00032 00033 char flash_buffer[2][FLASH_PAGE_SIZE]; 00034 int current_buffer; 00035 unsigned int flash_address; 00036 00037 /** flash_init 00038 */ 00039 void flash_init(void) { 00040 00041 /* Initialise the SSP0 to talk to the flash device. */ 00042 SSP0_init(); 00043 00044 DEBUG_INIT_START; 00045 00046 /* Clear out the page buffers. */ 00047 memset(flash_buffer, 0, 2 * FLASH_PAGE_SIZE); 00048 00049 /* Default the buffer in use. */ 00050 current_buffer = 0; 00051 00052 /* Default pointer set-up. */ 00053 flash_address = 0; 00054 00055 /* Prime our buffers for expected future access. */ 00056 flash_read_page(0, flash_buffer[0], true); 00057 flash_read_page(1, flash_buffer[1], true); 00058 00059 /* Although not part of the flash system, SOWB includes 00060 a 25AA02E48 device from Microchip that holds a globally 00061 unique 48bit (6byte) MAC address. We use this for the 00062 SOWB "STL authentic product" serial number and in future 00063 may be used as the ethernet MAC address if we ever write 00064 code to support Ethernet. This device is also connected 00065 to SSP1 and so we'll _init() it here now. */ 00066 //_25AA02E48_init(); 00067 00068 DEBUG_INIT_END; 00069 } 00070 00071 /** flash_process 00072 */ 00073 void flash_process(void) { 00074 /* Currently does nothing. */ 00075 } 00076 00077 /** flash_getc 00078 */ 00079 char flash_getc(bool peek) { 00080 char c; 00081 00082 /* Flash being deleted, undefined memory. */ 00083 if (sector_erase_in_progress) return 0xFF; 00084 00085 /* Wait for any page loads to complete. */ 00086 while (page_write_in_progress) user_call_process(); 00087 00088 /* Get the character from the internal buffer. */ 00089 c = flash_buffer[current_buffer][flash_address]; 00090 00091 /* If this is just a peek then return the character without 00092 incrementing the memory pointers etc etc. */ 00093 if (peek) return c; 00094 00095 /* Inc the address pointer and load a new page if needed. Note, 00096 we load the page in background using DMA. */ 00097 flash_address++; 00098 if ((flash_address & 0xFF) == 0) { 00099 flash_read_page(current_buffer >> 8, flash_buffer[current_buffer], false); 00100 current_buffer = current_buffer ? 0 : 1; 00101 } 00102 00103 return c; 00104 } 00105 00106 /** flash_seek 00107 */ 00108 void flash_seek(unsigned int addr) { 00109 flash_read_page((addr >> 8) + 0, flash_buffer[0], true); 00110 flash_read_page((addr >> 8) + 1, flash_buffer[1], true); 00111 flash_address = addr; 00112 }
Generated on Tue Jul 12 2022 18:05:35 by
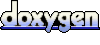