
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
diskio.h
00001 /*----------------------------------------------------------------------- 00002 / Low level disk interface modlue include file (C)ChaN, 2010 00003 /-----------------------------------------------------------------------*/ 00004 00005 #ifndef _DISKIO 00006 #define _DISKIO 00007 00008 #define _READONLY 0 /* 1: Remove write functions */ 00009 #define _USE_IOCTL 1 /* 1: Use disk_ioctl fucntion */ 00010 00011 #include "integer.h" 00012 00013 #ifdef __cplusplus 00014 extern "C" { 00015 #endif 00016 00017 /* Status of Disk Functions */ 00018 typedef BYTE DSTATUS; 00019 00020 /* Results of Disk Functions */ 00021 typedef enum { 00022 RES_OK = 0, /* 0: Successful */ 00023 RES_ERROR, /* 1: R/W Error */ 00024 RES_WRPRT, /* 2: Write Protected */ 00025 RES_NOTRDY, /* 3: Not Ready */ 00026 RES_PARERR /* 4: Invalid Parameter */ 00027 } DRESULT; 00028 00029 00030 /*---------------------------------------*/ 00031 /* Prototypes for disk control functions */ 00032 00033 int assign_drives (int, int); 00034 DSTATUS disk_initialize (BYTE); 00035 DSTATUS disk_status (BYTE); 00036 DRESULT disk_read (BYTE, BYTE*, DWORD, BYTE); 00037 DWORD get_fattime(void); 00038 #if _READONLY == 0 00039 DRESULT disk_write (BYTE, const BYTE*, DWORD, BYTE); 00040 #endif 00041 DRESULT disk_ioctl (BYTE, BYTE, void*); 00042 00043 00044 00045 /* Disk Status Bits (DSTATUS) */ 00046 00047 #define STA_NOINIT 0x01 /* Drive not initialized */ 00048 #define STA_NODISK 0x02 /* No medium in the drive */ 00049 #define STA_PROTECT 0x04 /* Write protected */ 00050 00051 00052 /* Command code for disk_ioctrl fucntion */ 00053 00054 /* Generic command (defined for FatFs) */ 00055 #define CTRL_SYNC 0 /* Flush disk cache (for write functions) */ 00056 #define GET_SECTOR_COUNT 1 /* Get media size (for only f_mkfs()) */ 00057 #define GET_SECTOR_SIZE 2 /* Get sector size (for multiple sector size (_MAX_SS >= 1024)) */ 00058 #define GET_BLOCK_SIZE 3 /* Get erase block size (for only f_mkfs()) */ 00059 #define CTRL_ERASE_SECTOR 4 /* Force erased a block of sectors (for only _USE_ERASE) */ 00060 00061 /* Generic command */ 00062 #define CTRL_POWER 5 /* Get/Set power status */ 00063 #define CTRL_LOCK 6 /* Lock/Unlock media removal */ 00064 #define CTRL_EJECT 7 /* Eject media */ 00065 00066 /* MMC/SDC specific ioctl command */ 00067 #define MMC_GET_TYPE 10 /* Get card type */ 00068 #define MMC_GET_CSD 11 /* Get CSD */ 00069 #define MMC_GET_CID 12 /* Get CID */ 00070 #define MMC_GET_OCR 13 /* Get OCR */ 00071 #define MMC_GET_SDSTAT 14 /* Get SD status */ 00072 00073 /* ATA/CF specific ioctl command */ 00074 #define ATA_GET_REV 20 /* Get F/W revision */ 00075 #define ATA_GET_MODEL 21 /* Get model name */ 00076 #define ATA_GET_SN 22 /* Get serial number */ 00077 00078 /* NAND specific ioctl command */ 00079 #define NAND_FORMAT 30 /* Create physical format */ 00080 00081 #ifdef __cplusplus 00082 } 00083 #endif 00084 00085 #endif
Generated on Tue Jul 12 2022 18:05:34 by
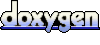