
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
debug_printf.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 /* The following is from (with only minor edits to fit onto Mbed):- 00024 Copyright 2001, 2002 Georges Menie (www.menie.org) 00025 stdarg version contributed by Christian Ettinger 00026 See... 00027 http://www.menie.org/georges/embedded/ 00028 http://www.menie.org/georges/embedded/printf-stdarg.c 00029 00030 This printf function will accept integer formats (%d, %x, %X, %u), string format (%s) 00031 and character format (%c); left and right alignement, padding with space or 'O'. */ 00032 00033 #include "sowb.h" 00034 #include "debug.h" 00035 #include <stdarg.h> 00036 00037 #ifdef DEBUG_ON 00038 00039 static void printchar(char **str, int c) 00040 { 00041 if (str) { 00042 **str = c; 00043 ++(*str); 00044 } 00045 else (void)Uart0_putc(c); 00046 } 00047 00048 #define PAD_RIGHT 1 00049 #define PAD_ZERO 2 00050 00051 static int prints(char **out, const char *string, int width, int pad) 00052 { 00053 register int pc = 0, padchar = ' '; 00054 00055 if (width > 0) { 00056 register int len = 0; 00057 register const char *ptr; 00058 for (ptr = string; *ptr; ++ptr) ++len; 00059 if (len >= width) width = 0; 00060 else width -= len; 00061 if (pad & PAD_ZERO) padchar = '0'; 00062 } 00063 if (!(pad & PAD_RIGHT)) { 00064 for ( ; width > 0; --width) { 00065 printchar (out, padchar); 00066 ++pc; 00067 } 00068 } 00069 for ( ; *string ; ++string) { 00070 printchar (out, *string); 00071 ++pc; 00072 } 00073 for ( ; width > 0; --width) { 00074 printchar (out, padchar); 00075 ++pc; 00076 } 00077 00078 return pc; 00079 } 00080 00081 /* the following should be enough for 32 bit int */ 00082 #define PRINT_BUF_LEN 12 00083 00084 static int printi(char **out, int i, int b, int sg, int width, int pad, int letbase) 00085 { 00086 char print_buf[PRINT_BUF_LEN]; 00087 register char *s; 00088 register int t, neg = 0, pc = 0; 00089 register unsigned int u = i; 00090 00091 if (i == 0) { 00092 print_buf[0] = '0'; 00093 print_buf[1] = '\0'; 00094 return prints (out, print_buf, width, pad); 00095 } 00096 00097 if (sg && b == 10 && i < 0) { 00098 neg = 1; 00099 u = -i; 00100 } 00101 00102 s = print_buf + PRINT_BUF_LEN-1; 00103 *s = '\0'; 00104 00105 while (u) { 00106 t = u % b; 00107 if( t >= 10 ) 00108 t += letbase - '0' - 10; 00109 *--s = t + '0'; 00110 u /= b; 00111 } 00112 00113 if (neg) { 00114 if( width && (pad & PAD_ZERO) ) { 00115 printchar (out, '-'); 00116 ++pc; 00117 --width; 00118 } 00119 else { 00120 *--s = '-'; 00121 } 00122 } 00123 00124 return pc + prints (out, s, width, pad); 00125 } 00126 00127 static int print(char **out, const char *format, va_list args ) 00128 { 00129 register int width, pad; 00130 register int pc = 0; 00131 char scr[2]; 00132 00133 for (; *format != 0; ++format) { 00134 if (*format == '%') { 00135 ++format; 00136 width = pad = 0; 00137 if (*format == '\0') break; 00138 if (*format == '%') goto out; 00139 if (*format == '-') { 00140 ++format; 00141 pad = PAD_RIGHT; 00142 } 00143 while (*format == '0') { 00144 ++format; 00145 pad |= PAD_ZERO; 00146 } 00147 for ( ; *format >= '0' && *format <= '9'; ++format) { 00148 width *= 10; 00149 width += *format - '0'; 00150 } 00151 if( *format == 's' ) { 00152 register char *s = (char *)va_arg( args, int ); 00153 pc += prints (out, s?s:"(null)", width, pad); 00154 continue; 00155 } 00156 if( *format == 'd' ) { 00157 pc += printi (out, va_arg( args, int ), 10, 1, width, pad, 'a'); 00158 continue; 00159 } 00160 if( *format == 'x' ) { 00161 pc += printi (out, va_arg( args, int ), 16, 0, width, pad, 'a'); 00162 continue; 00163 } 00164 if( *format == 'X' ) { 00165 pc += printi (out, va_arg( args, int ), 16, 0, width, pad, 'A'); 00166 continue; 00167 } 00168 if( *format == 'u' ) { 00169 pc += printi (out, va_arg( args, int ), 10, 0, width, pad, 'a'); 00170 continue; 00171 } 00172 if( *format == 'c' ) { 00173 /* char are converted to int then pushed on the stack */ 00174 scr[0] = (char)va_arg( args, int ); 00175 scr[1] = '\0'; 00176 pc += prints (out, scr, width, pad); 00177 continue; 00178 } 00179 } 00180 else { 00181 out: 00182 printchar (out, *format); 00183 ++pc; 00184 } 00185 } 00186 if (out) **out = '\0'; 00187 va_end( args ); 00188 return pc; 00189 } 00190 00191 00192 int debug_printf(const char *format, ...) { 00193 #ifdef DEBUG_ON 00194 va_list args; 00195 va_start( args, format ); 00196 return print( 0, format, args ); 00197 #else 00198 return 0; 00199 #endif 00200 } 00201 00202 int debug_sprintf(char *out, const char *format, ...) { 00203 #ifdef DEBUG_ON 00204 va_list args; 00205 va_start( args, format ); 00206 return print( &out, format, args ); 00207 #else 00208 return 0; 00209 #endif 00210 } 00211 00212 /* end of #ifdef DEBUG_ON */ 00213 #endif 00214
Generated on Tue Jul 12 2022 18:05:34 by
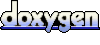