
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
debug.h
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef DEBUG_H 00024 #define DEBUG_H 00025 00026 /* Comment out the following to totally disable debugging output on UART0. 00027 This is the global kill switch for debug output. */ 00028 #define DEBUG_ON 00029 00030 00031 /* These are finer grained debug enable switches. */ 00032 #ifdef DEBUG_ON 00033 #define DEBUG_USE_UART0 00034 #endif 00035 00036 /* The following is used "interally" by the debug.c and various other modules. */ 00037 00038 void debug_init(void); 00039 00040 /* Buffer sizes MUST be a aligned 2^, for example 8, 16, 32, 64, 128, 256, 512, 1024, etc 00041 Do NOT use any other value or the buffer wrapping firmware won't work. */ 00042 #ifdef DEBUG_USE_UART0 00043 #define UART0_TX_BUFFER_SIZE 8192 00044 #define UART0_RX_BUFFER_SIZE 16 00045 #else 00046 #define UART0_TX_BUFFER_SIZE 4 00047 #define UART0_RX_BUFFER_SIZE 4 00048 #endif 00049 00050 00051 #ifdef DEBUG_USE_UART0 00052 /* If debugging is on declare the real function prototypes. */ 00053 int debug_printf(const char *format, ...); 00054 int debug_sprintf(char *out, const char *format, ...); 00055 void debug_string(char *s); 00056 void debug_stringl(char *s, int length); 00057 00058 #else 00059 00060 /* If no debugging, replace debug functions with simple empty macros. */ 00061 #define debug_printf(x, ...) 00062 #define debug_sprintf(x, y, ...) 00063 #define debug_string(x) 00064 #define debug_stringl(x, y) 00065 00066 /* End #ifdef DEBUG_USE_UART0 */ 00067 #endif 00068 00069 #define DEBUG_INIT_START debug_printf("INIT: %s()... ", __FUNCTION__) 00070 #define DEBUG_INIT_END debug_printf("complete.\r\n") 00071 00072 /* These function prototypes are always declared. */ 00073 void Uart0_putc(char c); 00074 int Uart0_getc(int block); 00075 00076 00077 /* End #ifndef DEBUG_H */ 00078 #endif 00079
Generated on Tue Jul 12 2022 18:05:34 by
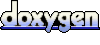