
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
config.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 /* Need to come back and finish this. */ 00024 00025 #include "sowb.h" 00026 #include "user.h" 00027 #include "debug.h" 00028 #include "config.h" 00029 #include "ff.h" 00030 00031 CONFIG_UNION system_config; 00032 00033 bool config_block_process; 00034 bool config_loaded; 00035 bool config_reload; 00036 00037 /** config_init 00038 */ 00039 void config_init(void) { 00040 int i, j; 00041 00042 DEBUG_INIT_START; 00043 00044 /* 00045 for (i = CONFIG_FLASH_PAGE_BASE, j = 0; i < 4096; i++, j++) { 00046 flash_read_page(i, system_config.buffers[j], true); 00047 } 00048 00049 config_loaded = true; 00050 config_reload = false; 00051 config_block_process = false; 00052 */ 00053 00054 DEBUG_INIT_END; 00055 } 00056 00057 /** config_process 00058 */ 00059 void config_process(void) { 00060 00061 /* For long period operations (e.g. config_save()) that may call 00062 system _process() functions, block ourselves from re-entering. */ 00063 if (config_block_process) return; 00064 00065 } 00066 00067 void config_save(void) { 00068 int i, j; 00069 char buffer[FLASH_PAGE_SIZE]; 00070 00071 /* Don't re-enter this function from _process(). */ 00072 config_block_process = true; 00073 00074 /* Sector 15 is used as a "scratch area". It allows us to store 00075 pages from other sectors that we need to "restore" since the 00076 LPC1768 doesn't have enough space to store entire sectors. */ 00077 while (flash_sector_erase_in_progress()) WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00078 flash_erase_sector(15); 00079 while (flash_sector_erase_in_progress()) WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00080 00081 /* We need to make a copy of all the pages below our config area 00082 before we store our configuration. */ 00083 for (i = 4096 - 256; i < CONFIG_FLASH_PAGE_BASE; i++) { 00084 flash_read_page(i, buffer, true); 00085 flash_page_write(3840 + i, buffer); 00086 while(flash_write_in_progress()) WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00087 } 00088 00089 /* Now erase the sector in which our config resides. */ 00090 while (flash_sector_erase_in_progress()) WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00091 flash_erase_sector(14); 00092 while (flash_sector_erase_in_progress()) WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00093 00094 00095 for (i = CONFIG_FLASH_PAGE_BASE, j = 0; i < CONFIG_FLASH_PAGE_BASE + CONFIG_FLASH_PAGES; i++, j++) { 00096 while(flash_write_in_progress() || flash_sector_erase_in_progress()) { 00097 WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00098 } 00099 flash_page_write(i, system_config.buffers[j]); 00100 } 00101 00102 config_block_process = false; 00103 } 00104 00105 /** config_copy_flash_page 00106 * 00107 * Used to copy the raw config struct, page by page 00108 * to an external memory buffer. 00109 * 00110 * @param int page The page to copy. 00111 * @param char* buffer The buffer to copy the page to. 00112 */ 00113 void config_copy_flash_page(int page, char *buffer) { 00114 memcpy(buffer, system_config.buffers[page], FLASH_PAGE_SIZE); 00115 } 00116 00117 /** config_get_page 00118 * 00119 * Get the base address of a specific page of config data. 00120 * 00121 * @param int page The page to get the address of. 00122 * @return char* The address of the page. 00123 */ 00124 char * config_get_page(int page) { 00125 return system_config.buffers[page]; 00126 } 00127 00128 00129
Generated on Tue Jul 12 2022 18:05:34 by
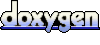