
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
MAX7456_chars.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "MAX7456_chars.h" 00024 00025 /* Why doesn't the MAX7456 have a + symbol? wtf! */ 00026 const unsigned char ascii_4d[54] = { 00027 0x55, 0x55, 0x55, 00028 0x55, 0x55, 0x55, 00029 0x55, 0x55, 0x55, 00030 0x55, 0x55, 0x55, 00031 0x55, 0x00, 0x55, 00032 0x55, 0x28, 0x55, 00033 0x55, 0x28, 0x55, 00034 0x00, 0x28, 0x00, 00035 0x4A, 0xAA, 0xA1, 00036 0x4A, 0xAA, 0xA1, 00037 0x00, 0x28, 0x00, 00038 0x55, 0x28, 0x55, 00039 0x55, 0x28, 0x55, 00040 0x55, 0x00, 0x55, 00041 0x55, 0x55, 0x55, 00042 0x55, 0x55, 0x55, 00043 0x55, 0x55, 0x55, 00044 0x55, 0x55, 0x55 }; 00045 00046 const unsigned char crosshair1_left[54] = { 00047 0x55, 0x55, 0x56, // 0 00048 0x55, 0x55, 0x56, // 1 00049 0x55, 0x55, 0x56, // 2 00050 0x55, 0x55, 0x56, // 3 00051 0x55, 0x55, 0x56, // 4 00052 0x55, 0x55, 0x56, // 5 00053 0x55, 0x55, 0x55, // 6 00054 0x55, 0x55, 0x55, // 7 00055 0x55, 0xAA, 0x95, // 8 00056 0x55, 0xAA, 0x95, // 9 - 8 00057 0x55, 0x55, 0x55, // 10 - 7 00058 0x55, 0x55, 0x55, // 11 - 6 00059 0x55, 0x55, 0x56, // 12 - 5 00060 0x55, 0x55, 0x56, // 13 - 4 00061 0x55, 0x55, 0x56, // 14 - 3 00062 0x55, 0x55, 0x56, // 15 - 2 00063 0x55, 0x55, 0x56, // 16 - 1 00064 0x55, 0x55, 0x56 }; 00065 00066 const unsigned char crosshair1_right[54] = { 00067 0x95, 0x55, 0x55, // 0 00068 0x95, 0x55, 0x55, // 1 00069 0x95, 0x55, 0x55, // 2 00070 0x95, 0x55, 0x55, // 3 00071 0x95, 0x55, 0x55, // 4 00072 0x95, 0x55, 0x55, // 5 00073 0x55, 0x55, 0x55, // 6 00074 0x55, 0x55, 0x55, // 7 00075 0x56, 0xAA, 0x55, // 8 00076 0x56, 0xAA, 0x55, // 9 - 8 00077 0x55, 0x55, 0x55, // 10 - 7 00078 0x55, 0x55, 0x55, // 11 - 6 00079 0x95, 0x55, 0x55, // 12 - 5 00080 0x95, 0x55, 0x55, // 13 - 4 00081 0x95, 0x55, 0x55, // 14 - 3 00082 0x95, 0x55, 0x55, // 15 - 2 00083 0x95, 0x55, 0x55, // 16 - 1 00084 0x95, 0x55, 0x55 }; 00085 00086 const unsigned char crosshair_centre[54] = { 00087 0x55, 0x28, 0x55, // 0 00088 0x55, 0x28, 0x55, // 1 00089 0x55, 0x28, 0x55, // 2 00090 0x55, 0x28, 0x55, // 3 00091 0x55, 0x28, 0x55, // 4 00092 0x55, 0x00, 0x55, // 5 00093 0x55, 0x55, 0x55, // 6 00094 0x01, 0x55, 0x40, // 7 00095 0xA1, 0x69, 0x4A, // 8 00096 0xA1, 0x69, 0x4A, // 9 00097 0x01, 0x55, 0x40, // 10 00098 0x55, 0x55, 0x55, // 11 00099 0x55, 0x00, 0x55, // 12 00100 0x55, 0x28, 0x55, // 13 00101 0x55, 0x28, 0x55, // 14 00102 0x55, 0x28, 0x55, // 15 00103 0x55, 0x28, 0x55, // 16 00104 0x55, 0x28, 0x55 }; 00105 00106 00107 const unsigned char ascii_b0[54] = { 00108 0x55, 0x55, 0x55, // 0 00109 0x55, 0x55, 0x55, // 1 00110 0x50, 0x01, 0x55, // 2 00111 0x42, 0xA0, 0x55, // 3 00112 0x0A, 0xA8, 0x15, // 4 00113 0x28, 0x0A, 0x15, // 5 00114 0x28, 0x0A, 0x15, // 6 00115 0x28, 0x0A, 0x15, 00116 0x0A, 0xA8, 0x15, 00117 0x42, 0xA0, 0x55, 00118 0x50, 0x01, 0x55, 00119 0x55, 0x55, 0x55, 00120 0x55, 0x55, 0x55, 00121 0x55, 0x55, 0x55, 00122 0x55, 0x55, 0x55, 00123 0x55, 0x55, 0x55, 00124 0x55, 0x55, 0x55, 00125 0x55, 0x55, 0x55 }; 00126 00127 const unsigned char ascii_b3[54] = { 00128 0x55, 0x28, 0x55, 00129 0x55, 0x28, 0x55, 00130 0x55, 0x28, 0x55, 00131 0x55, 0x28, 0x55, 00132 0x55, 0x28, 0x55, 00133 0x55, 0x28, 0x55, 00134 0x55, 0x28, 0x55, 00135 0x55, 0x28, 0x55, 00136 0x55, 0x28, 0x55, 00137 0x55, 0x28, 0x55, 00138 0x55, 0x28, 0x55, 00139 0x55, 0x28, 0x55, 00140 0x55, 0x28, 0x55, 00141 0x55, 0x28, 0x55, 00142 0x55, 0x28, 0x55, 00143 0x55, 0x28, 0x55, 00144 0x55, 0x28, 0x55, 00145 0x55, 0x28, 0x55 }; 00146 00147 const unsigned char ascii_b4[54] = { 00148 0x55, 0x28, 0x55, 00149 0x55, 0x28, 0x55, 00150 0x55, 0x28, 0x55, 00151 0x55, 0x28, 0x55, 00152 0x55, 0x28, 0x55, 00153 0x55, 0x28, 0x55, 00154 0x55, 0x28, 0x55, 00155 0x00, 0x28, 0x55, 00156 0xAA, 0xA8, 0x55, 00157 0xAA, 0xA8, 0x55, 00158 0x00, 0x28, 0x55, 00159 0x55, 0x28, 0x55, 00160 0x55, 0x28, 0x55, 00161 0x55, 0x28, 0x55, 00162 0x55, 0x28, 0x55, 00163 0x55, 0x28, 0x55, 00164 0x55, 0x28, 0x55, 00165 0x55, 0x28, 0x55 }; 00166 00167 const unsigned char ascii_bf[54] = { 00168 0x55, 0x55, 0x55, 00169 0x55, 0x55, 0x55, 00170 0x55, 0x55, 0x55, 00171 0x55, 0x55, 0x55, 00172 0x55, 0x55, 0x55, 00173 0x55, 0x55, 0x55, 00174 0x55, 0x55, 0x55, 00175 0x00, 0x00, 0x55, 00176 0xAA, 0xA8, 0x55, 00177 0xAA, 0xA8, 0x55, 00178 0x00, 0x28, 0x55, 00179 0x55, 0x28, 0x55, 00180 0x55, 0x28, 0x55, 00181 0x55, 0x28, 0x55, 00182 0x55, 0x28, 0x55, 00183 0x55, 0x28, 0x55, 00184 0x55, 0x28, 0x55, 00185 0x55, 0x28, 0x55 }; 00186 00187 const unsigned char ascii_c0[54] = { 00188 0x55, 0x28, 0x55, 00189 0x55, 0x28, 0x55, 00190 0x55, 0x28, 0x55, 00191 0x55, 0x28, 0x55, 00192 0x55, 0x28, 0x55, 00193 0x55, 0x28, 0x55, 00194 0x55, 0x28, 0x55, 00195 0x55, 0x28, 0x00, 00196 0x55, 0x2A, 0xAA, 00197 0x55, 0x2A, 0xAA, 00198 0x55, 0x00, 0x00, 00199 0x55, 0x55, 0x55, 00200 0x55, 0x55, 0x55, 00201 0x55, 0x55, 0x55, 00202 0x55, 0x55, 0x55, 00203 0x55, 0x55, 0x55, 00204 0x55, 0x55, 0x55, 00205 0x55, 0x55, 0x55 }; 00206 00207 const unsigned char ascii_c1[54] = { 00208 0x55, 0x28, 0x55, 00209 0x55, 0x28, 0x55, 00210 0x55, 0x28, 0x55, 00211 0x55, 0x28, 0x55, 00212 0x55, 0x28, 0x55, 00213 0x55, 0x28, 0x55, 00214 0x55, 0x28, 0x55, 00215 0x00, 0x28, 0x00, 00216 0xAA, 0xAA, 0xAA, 00217 0xAA, 0xAA, 0xAA, 00218 0x00, 0x00, 0x00, 00219 0x55, 0x55, 0x55, 00220 0x55, 0x55, 0x55, 00221 0x55, 0x55, 0x55, 00222 0x55, 0x55, 0x55, 00223 0x55, 0x55, 0x55, 00224 0x55, 0x55, 0x55, 00225 0x55, 0x55, 0x55 }; 00226 00227 const unsigned char ascii_c2[54] = { 00228 0x55, 0x55, 0x55, 00229 0x55, 0x55, 0x55, 00230 0x55, 0x55, 0x55, 00231 0x55, 0x55, 0x55, 00232 0x55, 0x55, 0x55, 00233 0x55, 0x55, 0x55, 00234 0x55, 0x55, 0x55, 00235 0x00, 0x00, 0x00, 00236 0xAA, 0xAA, 0xAA, 00237 0xAA, 0xAA, 0xAA, 00238 0x00, 0x28, 0x00, 00239 0x55, 0x28, 0x55, 00240 0x55, 0x28, 0x55, 00241 0x55, 0x28, 0x55, 00242 0x55, 0x28, 0x55, 00243 0x55, 0x28, 0x55, 00244 0x55, 0x28, 0x55, 00245 0x55, 0x28, 0x55 }; 00246 00247 const unsigned char ascii_c3[54] = { 00248 0x55, 0x28, 0x55, 00249 0x55, 0x28, 0x55, 00250 0x55, 0x28, 0x55, 00251 0x55, 0x28, 0x55, 00252 0x55, 0x28, 0x55, 00253 0x55, 0x28, 0x55, 00254 0x55, 0x28, 0x55, 00255 0x55, 0x28, 0x00, 00256 0x55, 0x2A, 0xAA, 00257 0x55, 0x2A, 0xAA, 00258 0x55, 0x28, 0x00, 00259 0x55, 0x28, 0x55, 00260 0x55, 0x28, 0x55, 00261 0x55, 0x28, 0x55, 00262 0x55, 0x28, 0x55, 00263 0x55, 0x28, 0x55, 00264 0x55, 0x28, 0x55, 00265 0x55, 0x28, 0x55 }; 00266 00267 const unsigned char ascii_c4[54] = { 00268 0x55, 0x55, 0x55, 00269 0x55, 0x55, 0x55, 00270 0x55, 0x55, 0x55, 00271 0x55, 0x55, 0x55, 00272 0x55, 0x55, 0x55, 00273 0x55, 0x55, 0x55, 00274 0x55, 0x55, 0x55, 00275 0x00, 0x00, 0x00, 00276 0xAA, 0xAA, 0xAA, 00277 0xAA, 0xAA, 0xAA, 00278 0x00, 0x00, 0x00, 00279 0x55, 0x55, 0x55, 00280 0x55, 0x55, 0x55, 00281 0x55, 0x55, 0x55, 00282 0x55, 0x55, 0x55, 00283 0x55, 0x55, 0x55, 00284 0x55, 0x55, 0x55, 00285 0x55, 0x55, 0x55 }; 00286 00287 const unsigned char ascii_c5[54] = { 00288 0x55, 0x28, 0x55, 00289 0x55, 0x28, 0x55, 00290 0x55, 0x28, 0x55, 00291 0x55, 0x28, 0x55, 00292 0x55, 0x28, 0x55, 00293 0x55, 0x28, 0x55, 00294 0x55, 0x28, 0x55, 00295 0x00, 0x28, 0x00, 00296 0xAA, 0xAA, 0xAA, 00297 0xAA, 0xAA, 0xAA, 00298 0x00, 0x28, 0x00, 00299 0x55, 0x28, 0x55, 00300 0x55, 0x28, 0x55, 00301 0x55, 0x28, 0x55, 00302 0x55, 0x28, 0x55, 00303 0x55, 0x28, 0x55, 00304 0x55, 0x28, 0x55, 00305 0x55, 0x28, 0x55 }; 00306 00307 const unsigned char ascii_d9[54] = { 00308 0x55, 0x28, 0x55, 00309 0x55, 0x28, 0x55, 00310 0x55, 0x28, 0x55, 00311 0x55, 0x28, 0x55, 00312 0x55, 0x28, 0x55, 00313 0x55, 0x28, 0x55, 00314 0x55, 0x28, 0x55, 00315 0x00, 0x28, 0x55, 00316 0xAA, 0xA8, 0x55, 00317 0xAA, 0xA8, 0x55, 00318 0x00, 0x00, 0x55, 00319 0x55, 0x55, 0x55, 00320 0x55, 0x55, 0x55, 00321 0x55, 0x55, 0x55, 00322 0x55, 0x55, 0x55, 00323 0x55, 0x55, 0x55, 00324 0x55, 0x55, 0x55, 00325 0x55, 0x55, 0x55 }; 00326 00327 const unsigned char ascii_da[54] = { 00328 0x55, 0x55, 0x55, 00329 0x55, 0x55, 0x55, 00330 0x55, 0x55, 0x55, 00331 0x55, 0x55, 0x55, 00332 0x55, 0x55, 0x55, 00333 0x55, 0x55, 0x55, 00334 0x55, 0x55, 0x55, 00335 0x55, 0x00, 0x00, 00336 0x55, 0x2A, 0xAA, 00337 0x55, 0x2A, 0xAA, 00338 0x55, 0x28, 0x00, 00339 0x55, 0x28, 0x55, 00340 0x55, 0x28, 0x55, 00341 0x55, 0x28, 0x55, 00342 0x55, 0x28, 0x55, 00343 0x55, 0x28, 0x55, 00344 0x55, 0x28, 0x55, 00345 0x55, 0x28, 0x55 }; 00346 00347 /* For the curious, during testing I managed to screw up the 00348 characher at CM index2, ('2'). So I needed to reprogram 00349 index2 with the correct character, doh! Anyway, since I 00350 went to the trouble I'll leave it in the code. I managed 00351 to find a default CM map at:- 00352 http://www.maxim-ic.com/tools/evkit/index.cfm?EVKit=558 00353 If you download this then be aware that the DEFAULTCM.MCM file 00354 defines each charmap as 64bytes, the 10 additional bytes need 00355 to be stripped off as "not used". */ 00356 #ifdef FIX_CM2_SCREW_UP 00357 const unsigned char ascii_02[54] = { 00358 0x55, 0x55, 0x55, // 01010101 01010101 01010101 00359 0x55, 0x55, 0x55, // 01010101 01010101 01010101 00360 0x54, 0x00, 0x15, // 01010100 00000000 00010101 00361 0x52, 0xAA, 0x85, // 01010010 10101010 10000101 00362 0x4A, 0xAA, 0xA1, // 01001010 10101010 10100001 00363 0x2A, 0x80, 0xA8, // 00101010 10000000 10101000 00364 0x2A, 0x15, 0x28, // 00101010 00010101 00101000 00365 0x40, 0x55, 0x28, // 01000000 01010101 00101000 00366 0x55, 0x54, 0xA8, // 01010101 01010100 10101000 00367 0x55, 0x52, 0xA1, // 01010101 01010010 10100001 00368 0x55, 0x4A, 0x85, // 01010101 01001010 10000101 00369 0x55, 0x2A, 0x15, // 01010101 00101010 00010101 00370 0x54, 0xA8, 0x55, // 01010100 10101000 01010101 00371 0x52, 0xA1, 0x55, // 01010010 10100001 01010101 00372 0x4A, 0x80, 0x01, // 01001010 10000000 00000001 00373 0x2A, 0xAA, 0xA8, // 00101010 10101010 10101000 00374 0x2A, 0xAA, 0xA8, // 00101010 10101010 10101000 00375 0x40, 0x00, 0x01 // 01000000 00000000 00000001 00376 }; 00377 #endif 00378 00379 /* Create an array of structures that link the data 00380 characters to ASCII characters. The last entry 00381 must always be null so we can detect the end of 00382 the array. */ 00383 MAX7456_CUSTOM_CHAR custom_chars[] = { 00384 { 0xE0, crosshair1_left }, 00385 { 0xE1, crosshair1_right }, 00386 { 0xE2, crosshair_centre }, 00387 { 0x4D, ascii_4d }, 00388 { 0xB0, ascii_b0 }, 00389 { 0xB3, ascii_b3 }, 00390 { 0xB4, ascii_b4 }, 00391 { 0xBF, ascii_bf }, 00392 { 0xC0, ascii_c0 }, 00393 { 0xC1, ascii_c1 }, 00394 { 0xC2, ascii_c2 }, 00395 { 0xC3, ascii_c3 }, 00396 { 0xC4, ascii_c4 }, 00397 { 0xC5, ascii_c5 }, 00398 { 0xD9, ascii_d9 }, 00399 { 0xDA, ascii_da }, 00400 #ifdef FIX_CM2_SCREW_UP 00401 { 0x02, ascii_02 }, 00402 #endif 00403 { 0x00, 0 } 00404 }; 00405
Generated on Tue Jul 12 2022 18:05:35 by
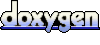