
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
25AA02EE48.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "sowb.h" 00024 #include "user.h" 00025 #include "flash.h" 00026 #include "ssp0.h" 00027 #include "gpio.h" 00028 00029 char mac_addr[6]; 00030 00031 /** _25AA02E48_mac_addr 00032 * 00033 * Get a copy of the MAC address with a null terminator. 00034 * 00035 * @param char *s a buffer, 7 bytes long, to hold the MAC+null 00036 */ 00037 void _25AA02E48_mac_addr(char *s) { 00038 memcpy(s, mac_addr, 6); 00039 s[6] = '\0'; 00040 } 00041 00042 /** _25AA02E48_mac_addr_printable 00043 * 00044 * Create a string that represents the MAC addr as a 00045 * printable ASCII string. The caller is responsible 00046 * for allocating enough space in the buffer pointed 00047 * to by s to hold the string. 00048 * 00049 * @param char *s a buffer, 18 bytes long, to hold the MAC 00050 * @param char divider A character to divide the bytes or 0 00051 */ 00052 void _25AA02E48_mac_addr_printable(char *s, char divider) { 00053 if (divider != 0) { 00054 sprintf(s, "%02X%c%02X%c%02X%c%02X%c%02X%c%02X", 00055 mac_addr[0], divider, mac_addr[1], divider, mac_addr[2], divider, 00056 mac_addr[3], divider, mac_addr[4], divider, mac_addr[5]); 00057 } 00058 else { 00059 sprintf(s, "%02X%02X%02X%02X%02X%02X", 00060 mac_addr[0], mac_addr[1], mac_addr[2], 00061 mac_addr[3], mac_addr[4], mac_addr[5]); 00062 } 00063 } 00064 00065 /** _25AA02E48_init 00066 */ 00067 void _25AA02E48_init(void) { 00068 00069 /* Assumes SSP0 is already _init() */ 00070 00071 while(!SSP0_request()) WHILE_WAITING_DO_PROCESS_FUNCTIONS; 00072 00073 LPC_SSP0->CPSR = _25AA02E48_SSP_INIT_CPSR; 00074 AA02E48_CS_ASSERT; 00075 FLASH_SHORT_COMMAND(FLASH_READ); 00076 SSP0_WRITE_BYTE(0xFA); 00077 SSP0_FLUSH_RX_FIFO; 00078 00079 for (int i = 0; i < 6; i++) { 00080 SSP0_WRITE_BYTE(0x00); 00081 while(SSP0_IS_BUSY || (LPC_SSP0->SR & (1UL << 2)) == 0); 00082 mac_addr[i] = (char)LPC_SSP0->DR; 00083 } 00084 00085 AA02E48_CS_DEASSERT; 00086 LPC_SSP0->CPSR = FLASH_SSP_INIT_CPSR; 00087 SSP0_release(); 00088 }
Generated on Tue Jul 12 2022 18:05:34 by
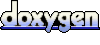