This module provides a simple API to the Maxim MAX7456 on-screen display chip
Embed:
(wiki syntax)
Show/hide line numbers
OSD7456.cpp
00001 /* 00002 Copyright (c) 2010 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #include "mbed.h" 00024 #include "OSD7456.h" 00025 00026 void 00027 OSD7456::init(void) 00028 { 00029 for (int i = 0; i < OSD_NUM_LINES; i++) { clear(i); } 00030 oddEven = false; 00031 max7456->vsync_set_callback(this, &OSD7456::vsync); 00032 } 00033 00034 void 00035 OSD7456::clear(int line) 00036 { 00037 lines[line].updated = true; 00038 memset(lines[line].line, ' ', OSD_LINE_LEN); 00039 memset(lines[line].attrib, 0, OSD_LINE_LEN); 00040 } 00041 00042 void 00043 OSD7456::vsync(void) 00044 { 00045 if (oddEven) { 00046 oddEven = false; 00047 } 00048 else { 00049 for (int i = 0; i < OSD_NUM_LINES; i++) { 00050 if (lines[i].updated) { 00051 max7456->stringxy(0, i, lines[i].line, lines[i].attrib); 00052 lines[i].updated = false; 00053 } 00054 } 00055 oddEven = true; 00056 cb_vsync.call(); 00057 } 00058 } 00059 00060 int 00061 OSD7456::print(int line, char *s) 00062 { 00063 int i = 0; 00064 while (*s != '\0') { 00065 lines[line].line[i] = *s; 00066 s++; i++; 00067 } 00068 lines[line].updated = true; 00069 return i; 00070 } 00071 00072 int 00073 OSD7456::print(int line, char *s, char attrib) 00074 { 00075 int i = 0; 00076 while (*s != '\0') { 00077 lines[line].line[i] = *s; 00078 lines[line].attrib[i] = attrib; 00079 s++; i++; 00080 } 00081 lines[line].updated = true; 00082 return i; 00083 } 00084 00085 int 00086 OSD7456::print(int x, int y, char *s) 00087 { 00088 int i = 0; 00089 while (*s != '\0') { 00090 lines[y].line[i+x] = *s; 00091 s++; i++; 00092 } 00093 lines[y].updated = true; 00094 return i; 00095 } 00096 00097 int 00098 OSD7456::print(int x, int y, char *s, char attrib) 00099 { 00100 int i = 0; 00101 while (*s != '\0') { 00102 lines[y].line[i+x] = *s; 00103 lines[y].attrib[i+x] = attrib; 00104 s++; i++; 00105 } 00106 lines[y].updated = true; 00107 return i; 00108 }
Generated on Wed Jul 13 2022 11:53:43 by
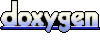