
Shiftbrite sequencer demo program. Controls a chain of 35 shiftbrites
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 // Shiftbrite Sequencer Demo Program 00003 // 00004 //Bits for Shiftbrite latch and enable pins 00005 DigitalOut latch(p15); 00006 DigitalOut enable(p16); 00007 //DigitalOut myled(LED1); 00008 00009 // SPI connections for LED chain 00010 SPI spi(p11, p12, p13); 00011 00012 //Serial PC(USBTX, USBRX); 00013 00014 Timer time_counter; 00015 00016 // number of LEDS in chain 00017 #define num_LEDS 35 00018 00019 // number of color values to sequence through 00020 #define Sequence_Length 17 00021 00022 // number of steps in fade effect 00023 #define Fade_Steps 64 00024 00025 00026 // Each LED'S RGB color values for each step in sequence is stored in an array 00027 int LED_Color [Sequence_Length][num_LEDS][3] = {0}; 00028 00029 // delay times in seconds for each RGB color value in sequence 00030 // can be different for each sequence step 00031 float Sequence_Delay[Sequence_Length]; 00032 int Sequence_Effect[Sequence_Length] = {0}; 00033 00034 00035 // Write (Shift out) 10-bit RGB values to a single LED 00036 void Write_LED(int red, int green, int blue) { 00037 unsigned int low_color=0; 00038 unsigned int high_color=0; 00039 red=red; 00040 green=green; 00041 blue=blue; 00042 high_color=(blue<<4)|((red&0x3C0)>>6); 00043 low_color=(((red&0x3F)<<10)|(green)); 00044 spi.write(high_color); 00045 spi.write(low_color); 00046 } 00047 // Sends Initial Current limits to all LEDs in array 00048 // used to correct for different RGB brightness levels in LEDs 00049 void Write_Init_Command(int red_level, int green_level, int blue_level) { 00050 unsigned int Init_Command = 0x40000000; 00051 int i=0; 00052 Init_Command = 0x40000000|(blue_level<<20)|(red_level<<10)|green_level; 00053 for (i=0; i<num_LEDS; i++) { 00054 spi.write(Init_Command>>16&0xFFFF); 00055 spi.write(Init_Command&0xFFFF); 00056 } 00057 wait(.000015); 00058 latch=1; 00059 wait(.000015); 00060 latch=0; 00061 } 00062 00063 // Sets all LEDs in a sequence step to a default background color 00064 void Set_Background_Color(int Seq_Step, int red, int green, int blue) { 00065 int i=0; 00066 for (i=0; i<num_LEDS; i++) { 00067 LED_Color[Seq_Step][i][0] = red; 00068 LED_Color[Seq_Step][i][1] = green; 00069 LED_Color[Seq_Step][i][2] = blue; 00070 } 00071 } 00072 00073 // Set a single LED in a Sequence Step to a color 00074 // Last LED in chain is LED #0 00075 // 00076 // LED address in 5x7 array 00077 // 35 in chain serpantine style 00078 // 34 33 32 31 30 29 28 00079 // 27 26 25 24 23 22 21 00080 // 20 19 18 17 16 15 14 00081 // 13 12 11 10 09 08 07 00082 // 06 05 04 03 02 01 00 00083 void Set_LED_Color(int Seq_Step, int LED_num, int red, int green, int blue) { 00084 LED_Color[Seq_Step][LED_num][0] = red; 00085 LED_Color[Seq_Step][LED_num][1] = green; 00086 LED_Color[Seq_Step][LED_num][2] = blue; 00087 } 00088 00089 int main() { 00090 int i=0; 00091 int j=0; 00092 int k=0; 00093 int red, green, blue; 00094 bool odd=false; 00095 spi.format(16,0); 00096 spi.frequency(500000); 00097 enable=0; 00098 latch=0; 00099 int flicker = 0; 00100 // Set currents using Allegro A6281's command mode 00101 Write_Init_Command(120, 100, 100); 00102 // Do once during initialization 00103 // OK at power on, but a reset could set it to an invalid mode 00104 // 00105 // See page 7 in A6281 datasheet 00106 // This feature can be used to adjust for different LED 00107 // brightness levels - values suggested from Macetech doc 00108 00109 // Clear out initial sequence values 00110 for (i=0; i<Sequence_Length; i++) { 00111 for (j=0; j<num_LEDS; j++) { 00112 for (k=0; k<4; k++) { 00113 LED_Color[i][j][k]=0; 00114 } 00115 } 00116 Sequence_Delay[i]=0; 00117 Sequence_Effect[i]=0; 00118 } 00119 // Color data values for LEDs to sequence through 00120 // Sequence step 0 00121 Set_Background_Color(0, 1023, 0, 0); //LEDs RED 00122 Sequence_Delay[0] = 1; 00123 Sequence_Effect[0] = 0; 00124 // Sequence step 1 00125 LED_Color[1][0][0] = 0; 00126 Set_Background_Color(1, 0, 1023, 0); //LEDs GREEN 00127 Sequence_Delay[1] = 2; 00128 Sequence_Effect[1] = 1; //Fade from RED to GREEN Effect 00129 // Sequence step 2 00130 Set_Background_Color(2, 0, 0, 1023); //LEDs BLUE 00131 Sequence_Delay[2] = 2; 00132 Sequence_Effect[2] = 1; //Fade from GREEN to BLUE Effect 00133 // Sequence step 3 00134 Set_Background_Color(3, 0, 0, 1023); 00135 Sequence_Delay[3] = 1; //Stay at this value for 1 seconds 00136 Sequence_Effect[3] = 0; 00137 // Sequence step 4 00138 Set_Background_Color(4,1023,200,0); //LEDs all ORANGE 00139 Sequence_Delay[4] = 5; 00140 Sequence_Effect[4] = 2; //Flicker or Twinkle Effect 00141 // Sequence step 5 00142 Set_Background_Color(5,1023,1023,0); //LEDs all YELLOW 00143 Sequence_Delay[5] = 5; 00144 Sequence_Effect[5] = 3; //Flashing Marquee Sign Effect 00145 // Sequence step 6 00146 LED_Color[6][0][0] = 1023; //LED 0 RED 00147 LED_Color[6][0][1] = 0; 00148 LED_Color[6][0][2] = 0; 00149 LED_Color[6][1][0] = 1023; 00150 LED_Color[6][1][1] = 1023; 00151 LED_Color[6][1][2] = 1023; //LED 1 WHITE 00152 LED_Color[6][2][0] = 0; 00153 LED_Color[6][2][1] = 0; 00154 LED_Color[6][2][2] = 1023; //LED 1 BLUE 00155 Sequence_Delay[6] = 5; 00156 Sequence_Effect[6] = 4; //Shift Colors 00157 // Sequence step 7 00158 Set_Background_Color(7,1023,1023,1023); //LEDs all WHITE 00159 Sequence_Delay[7] = 1; 00160 Sequence_Effect[7] = 0; 00161 //Sequence step 8 00162 Set_Background_Color(8,0,0,0); //LEDs all Black 00163 Sequence_Delay[8] = 2; 00164 Sequence_Effect[8]= 1; //Fade to Black 00165 //Sequence step 9 00166 Set_Background_Color(9,0,0,0); //LEDs all Black 00167 Sequence_Delay[9] = 1.5; 00168 Sequence_Effect[9]= 0; 00169 //Sequence step 10 00170 Sequence_Delay[10] = 6; 00171 Sequence_Effect[10]= 5; //Random Bright Colors 00172 //Sequence step 11 00173 Set_Background_Color(11,100,100,100); //LEDs all dim WHITE 00174 Set_LED_Color(11,34,0,0,1023); //char M 00175 Set_LED_Color(11,33,0,0,1023); 00176 Set_LED_Color(11,32,0,0,1023); 00177 Set_LED_Color(11,31,0,0,1023); 00178 Set_LED_Color(11,30,0,0,1023); 00179 Set_LED_Color(11,29,0,0,1023); 00180 Set_LED_Color(11,28,0,0,1023); 00181 Set_LED_Color(11,22,0,0,1023); 00182 Set_LED_Color(11,16,0,0,1023); 00183 Set_LED_Color(11,17,0,0,1023); 00184 Set_LED_Color(11,8,0,0,1023); 00185 Set_LED_Color(11,0,0,0,1023); 00186 Set_LED_Color(11,1,0,0,1023); 00187 Set_LED_Color(11,2,0,0,1023); 00188 Set_LED_Color(11,3,0,0,1023); 00189 Set_LED_Color(11,4,0,0,1023); 00190 Set_LED_Color(11,5,0,0,1023); 00191 Set_LED_Color(11,6,0,0,1023); 00192 Sequence_Effect[11] = 0; 00193 Sequence_Delay[11] = 3; 00194 //Sequence step 12 00195 Set_Background_Color(12,100,100,100); //LEDs all dim WHITE 00196 Set_LED_Color(12,34,0,0,1023); // char b 00197 Set_LED_Color(12,33,0,0,1023); 00198 Set_LED_Color(12,32,0,0,1023); 00199 Set_LED_Color(12,31,0,0,1023); 00200 Set_LED_Color(12,30,0,0,1023); 00201 Set_LED_Color(12,29,0,0,1023); 00202 Set_LED_Color(12,28,0,0,1023); 00203 Set_LED_Color(12,27,0,0,1023); 00204 Set_LED_Color(12,24,0,0,1023); 00205 Set_LED_Color(12,20,0,0,1023); 00206 Set_LED_Color(12,17,0,0,1023); 00207 Set_LED_Color(12,12,0,0,1023); 00208 Set_LED_Color(12,11,0,0,1023); 00209 Sequence_Effect[12] = 0; 00210 Sequence_Delay[12] = 3; 00211 //Sequence step 13 00212 Set_Background_Color(13,100,100,100); //LEDs all dim WHITE 00213 Set_LED_Color(13,34,0,0,1023); //char E 00214 Set_LED_Color(13,33,0,0,1023); 00215 Set_LED_Color(13,32,0,0,1023); 00216 Set_LED_Color(13,31,0,0,1023); 00217 Set_LED_Color(13,30,0,0,1023); 00218 Set_LED_Color(13,29,0,0,1023); 00219 Set_LED_Color(13,28,0,0,1023); 00220 Set_LED_Color(13,27,0,0,1023); 00221 Set_LED_Color(13,24,0,0,1023); 00222 Set_LED_Color(13,21,0,0,1023); 00223 Set_LED_Color(13,20,0,0,1023); 00224 Set_LED_Color(13,17,0,0,1023); 00225 Set_LED_Color(13,14,0,0,1023); 00226 Set_LED_Color(13,13,0,0,1023); 00227 Set_LED_Color(13,7,0,0,1023); 00228 Set_LED_Color(13,6,0,0,1023); 00229 Set_LED_Color(13,0,0,0,1023); 00230 Sequence_Effect[13] = 0; 00231 Sequence_Delay[13] = 3; 00232 //Sequence step 14 00233 Set_Background_Color(14,100,100,100); //LEDs all dim WHITE 00234 Set_LED_Color(14,5,0,0,1023); //char d 00235 Set_LED_Color(14,4,0,0,1023); 00236 Set_LED_Color(14,3,0,0,1023); 00237 Set_LED_Color(14,6,0,0,1023); 00238 Set_LED_Color(14,2,0,0,1023); 00239 Set_LED_Color(14,1,0,0,1023); 00240 Set_LED_Color(14,0,0,0,1023); 00241 Set_LED_Color(14,13,0,0,1023); 00242 Set_LED_Color(14,10,0,0,1023); 00243 Set_LED_Color(14,20,0,0,1023); 00244 Set_LED_Color(14,17,0,0,1023); 00245 Set_LED_Color(14,26,0,0,1023); 00246 Set_LED_Color(14,25,0,0,1023); 00247 Sequence_Effect[14] = 0; 00248 Sequence_Delay[14] = 3; 00249 //Sequence step 15 00250 Set_Background_Color(15,0,0,0); //LEDs all Black 00251 Sequence_Delay[15] = 2; 00252 Sequence_Effect[15]= 1; //Fade to Black 00253 //Sequence step 16 00254 Set_Background_Color(16,0,0,0); //LEDs all Black 00255 Sequence_Delay[16] = 2; 00256 Sequence_Effect[16]= 0; //Black 00257 // add more steps or LEDs if needed and change #defines 00258 00259 wait(1); 00260 // Repeat Sequence Forever 00261 while (1) { 00262 Write_Init_Command(120, 100, 100); 00263 //Step through the Sequence Values 00264 for (j = 0; j < Sequence_Length; j++) { 00265 // myled= !myled; 00266 switch (Sequence_Effect[j]) { 00267 case 0: { 00268 // Step through each LED in chain at each sequence step 00269 for (i = 0; i < num_LEDS; i++) { 00270 //Writes 32-bits of RGB color data to LED using SPI hardware 00271 Write_LED( LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00272 // Uncomment to get status info on serial port 00273 // printf("i= %d, j= %d\n\r",i,j); 00274 // printf(" %d %d %d\n\r",LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00275 } 00276 //Load in new values just shifted out to LED chain by setting latch high 00277 wait(.000015); 00278 latch=1; 00279 wait(.000015); 00280 latch=0; 00281 //Delay for this step in the sequence 00282 wait(Sequence_Delay[j]); 00283 break; 00284 } 00285 case 1: { 00286 //Lighing Effect: Fade into new color from previous color (note: can't fade first sequence step!) 00287 for (k = 0; k<=Fade_Steps; k++) { 00288 // Step through each LED in chain at each fade sequence step 00289 for (i = 0; i < num_LEDS; i++) { 00290 red = LED_Color[j-1][i][0] + k*(LED_Color[j][i][0]-LED_Color[j-1][i][0])/Fade_Steps; 00291 green = LED_Color[j-1][i][1] + k*(LED_Color[j][i][1]-LED_Color[j-1][i][1])/Fade_Steps; 00292 blue = LED_Color[j-1][i][2] + k*(LED_Color[j][i][2]-LED_Color[j-1][i][2])/Fade_Steps; 00293 //Writes 32-bits of RGB color data to LED using SPI hardware 00294 Write_LED(red, green, blue); 00295 // Uncomment to get status info on serial port 00296 // printf("i= %d, j= %d\n\r",i,j); 00297 // printf(" %d %d %d\n\r",LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00298 } 00299 //Load in new values just shifted out to LED chain by setting latch high 00300 wait(.000015); 00301 latch=1; 00302 wait(.000015); 00303 latch=0; 00304 //Delay for this step in the fade sequence 00305 wait(Sequence_Delay[j]/Fade_Steps); 00306 } 00307 break; 00308 } 00309 case 2: { 00310 //Lighting Effect: Flicker or Twinkle somewhat like a flame 00311 time_counter.reset(); 00312 time_counter.start(); 00313 while (time_counter.read()<Sequence_Delay[j]) { 00314 for (i = 0; i < num_LEDS; i++) { 00315 flicker = rand(); 00316 if (flicker>0x40000000) 00317 //Writes 32-bits of RGB color data to LED using SPI hardware 00318 Write_LED( LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00319 // Uncomment to get status info on serial port 00320 // printf("i= %d, j= %d\n\r",i,j); 00321 // printf(" %d %d %d\n\r",LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00322 else 00323 Write_LED( LED_Color[j][i][0]/2, LED_Color[j][i][1]/2, LED_Color[j][i][2]/2); 00324 } 00325 //Load in new values just shifted out to LED chain by setting latch high 00326 wait(.000015); 00327 latch=1; 00328 wait(.000015); 00329 latch=0; 00330 wait(.05); 00331 } 00332 time_counter.stop(); 00333 break; 00334 } 00335 case 3: { 00336 //Lighting Effect: Flashing Broadway Marquee Sign Style 00337 time_counter.reset(); 00338 time_counter.start(); 00339 while (time_counter.read()<Sequence_Delay[j]) { 00340 for (i = 0; i < num_LEDS; i++) { 00341 if (((i+odd)&0x01)==0) 00342 //Writes 32-bits of RGB color data to LED using SPI hardware 00343 Write_LED( LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00344 // Uncomment to get status info on serial port 00345 // printf("i= %d, j= %d\n\r",i,j); 00346 // printf(" %d %d %d\n\r",LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00347 else 00348 Write_LED( LED_Color[j][i][0]/4, LED_Color[j][i][1]/4, LED_Color[j][i][2]/4); 00349 } 00350 //Load in new values just shifted out to LED chain by setting latch high 00351 wait(.000015); 00352 latch=1; 00353 wait(.000015); 00354 latch=0; 00355 wait(.15); 00356 odd = !odd; 00357 } 00358 time_counter.stop(); 00359 break; 00360 } 00361 case 4: { 00362 //Lighting Effect: Circular Shift of Colors 00363 time_counter.reset(); 00364 time_counter.start(); 00365 k=0; 00366 while (time_counter.read()<Sequence_Delay[j]) { 00367 k = (k+1)%num_LEDS; 00368 for (i = 0; i < num_LEDS; i++) { 00369 00370 //Writes 32-bits of RGB color data to LED using SPI hardware 00371 Write_LED( LED_Color[j][(i+k)%num_LEDS][0], LED_Color[j][(i+k)%num_LEDS][1], LED_Color[j][(i+k)%num_LEDS][2]); 00372 // Uncomment to get status info on serial port 00373 // printf("i= %d, j= %d\n\r",i,j); 00374 // printf(" %d %d %d\n\r",LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00375 } 00376 //Load in new values just shifted out to LED chain by setting latch high 00377 wait(.000015); 00378 latch=1; 00379 wait(.000015); 00380 latch=0; 00381 wait(.10); 00382 } 00383 time_counter.stop(); 00384 break; 00385 case 5: { 00386 //Random Bright Colors 00387 time_counter.reset(); 00388 time_counter.start(); 00389 k=0; 00390 while (time_counter.read()<Sequence_Delay[j]) { 00391 k = (k+1)%num_LEDS; 00392 for (i = 0; i < num_LEDS; i++) { 00393 00394 //Writes 32-bits of RGB color data to LED using SPI hardware 00395 Write_LED( (rand()&0x00F000)>>6,(rand()&0x00F000)>>6,(rand()&0x00F000)>>6); 00396 // Uncomment to get status info on serial port 00397 // printf("i= %d, j= %d\n\r",i,j); 00398 // printf(" %d %d %d\n\r",LED_Color[j][i][0], LED_Color[j][i][1], LED_Color[j][i][2]); 00399 } 00400 //Load in new values just shifted out to LED chain by setting latch high 00401 wait(.000015); 00402 latch=1; 00403 wait(.000015); 00404 latch=0; 00405 wait(.08); 00406 } 00407 time_counter.stop(); 00408 break; 00409 } 00410 } 00411 } 00412 } 00413 } 00414 }
Generated on Sun Jul 17 2022 06:14:01 by
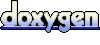