A library for talking to Multi-Tech's Cellular SocketModem Devices.
Dependents: M2X_dev axeda_wrapper_dev MTS_M2x_Example1 MTS_Cellular_Connect_Example ... more
MTSSerialFlowControl.h
00001 /* Universal Socket Modem Interface Library 00002 * Copyright (c) 2013 Multi-Tech Systems 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MTSSERIALFLOWCONTROL_H 00018 #define MTSSERIALFLOWCONTROL_H 00019 00020 #include "mbed.h" 00021 #include "MTSSerial.h" 00022 00023 00024 namespace mts 00025 { 00026 00027 /** This class derives from MTSBufferedIO/MTSSerial and provides a buffered wrapper to the 00028 * standard mbed Serial class along with generic RTS/CTS HW flow control. Since it 00029 * depends only on the mbed Serial, DigitalOut and InterruptIn classes for accessing 00030 * the serial data, this class is inherently portable accross different mbed platforms 00031 * and provides HW flow control even when not natively supported by the processors 00032 * serial port. If HW flow control is not needed, use MTSSerial instead. It should also 00033 * be noted that the RTS/CTS functionality in this class is implemented as a DTE device. 00034 */ 00035 class MTSSerialFlowControl : public MTSSerial 00036 { 00037 public: 00038 /** Creates a new MTSSerialFlowControl object that can be used to talk to an mbed serial 00039 * port through internal SW buffers. Note that this class also adds the ability to use 00040 * RTS/CTS HW Flow Conrtol through and standard mbed DigitalIn and DigitalOut pins. 00041 * The RTS and CTS functionality assumes this is a DTE device. 00042 * 00043 * @param TXD the transmit data pin on the desired mbed serial interface. 00044 * @param RXD the receive data pin on the desired mbed serial interface. 00045 * @param RTS the DigitalOut pin that RTS will be attached to. (DTE) 00046 * @param CTS the DigitalIn pin that CTS will be attached to. (DTE) 00047 * @param txBufferSize the size in bytes of the internal SW transmit buffer. The 00048 * default is 64 bytes. 00049 * @param rxBufferSize the size in bytes of the internal SW receive buffer. The 00050 * default is 64 bytes. 00051 * @param name an optional name for the serial port. The default is blank. 00052 */ 00053 MTSSerialFlowControl(PinName TXD, PinName RXD, PinName RTS, PinName CTS, int txBufSize = 64, int rxBufSize = 64); 00054 00055 /** Destructs an MTSSerialFlowControl object and frees all related resources, 00056 * including internal buffers. 00057 */ 00058 ~MTSSerialFlowControl(); 00059 00060 /** This method clears all the data from the internal Rx or read buffer. 00061 */ 00062 virtual void rxClear(); 00063 00064 private: 00065 void notifyStartSending(); // Used to set cts start signal 00066 void notifyStopSending(); // Used to set cts stop signal 00067 00068 //This device acts as a DTE 00069 bool rxReadyFlag; //Tracks state change for rts signaling 00070 DigitalOut rts; // Used to tell DCE to send or not send data 00071 DigitalIn cts; // Used to check if DCE is ready for data 00072 int highThreshold; // High water mark for setting cts to stop 00073 int lowThreshold; // Low water mark for setting cts to start 00074 00075 virtual void handleRead(); // Method for handling data to be read 00076 virtual void handleWrite(); // Method for handling data to be written 00077 }; 00078 00079 } 00080 00081 #endif /* MTSSERIALFLOWCONTROL */
Generated on Tue Jul 12 2022 21:46:23 by
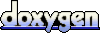