A library for talking to Multi-Tech's Cellular SocketModem Devices.
Dependents: M2X_dev axeda_wrapper_dev MTS_M2x_Example1 MTS_Cellular_Connect_Example ... more
MTSBufferedIO.h
00001 /* Universal Socket Modem Interface Library 00002 * Copyright (c) 2013 Multi-Tech Systems 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MTSBUFFEREDIO_H 00018 #define MTSBUFFEREDIO_H 00019 00020 #include "mbed.h" 00021 #include "MTSCircularBuffer.h" 00022 00023 namespace mts { 00024 00025 /** This is an abstract class for lightweight buffered io to an underlying 00026 * data interface. Specifically the inheriting class will need to override 00027 * both the handleRead and handleWrite methods which transfer data between 00028 * the class's internal read and write buffers and the physical communications 00029 * link or its HW buffers. 00030 */ 00031 00032 class MTSBufferedIO 00033 { 00034 public: 00035 /** Creates a new BufferedIO object with the passed in static buffer sizes. 00036 * Note that because this class is abstract you cannot construct it directly. 00037 * Instead, please construct one of its derived classes like MTSSerial or 00038 * MTSSerialFlowControl. 00039 * 00040 * @param txBufferSize the size of the Tx or write buffer in bytes. The default is 00041 * 128 bytes. 00042 * @param rxBufferSize the size of the Rx or read buffer in bytes. The default is 00043 * 128 bytes. 00044 */ 00045 MTSBufferedIO(int txBufferSize = 128, int rxBufferSize = 128); 00046 00047 /** Destructs an MTSBufferedIO object and frees all related resources, including 00048 * internal buffers. 00049 */ 00050 ~MTSBufferedIO(); 00051 00052 /** This method enables bulk writes to the Tx or write buffer. If more data 00053 * is requested to be written then space available the method writes 00054 * as much data as possible within the timeout period and returns the actual amount written. 00055 * 00056 * @param data the byte array to be written. 00057 * @param length the length of data to be written from the data parameter. 00058 * @timeoutMillis amount of time in milliseconds to complete operation. 00059 * @returns the number of bytes written to the buffer, which is 0 if 00060 * the buffer is full. 00061 */ 00062 int write(const char* data, int length, unsigned int timeoutMillis); 00063 00064 /** This method enables bulk writes to the Tx or write buffer. If more data 00065 * is requested to be written then space available the method writes 00066 * as much data as possible and returns the actual amount written. 00067 * 00068 * @param data the byte array to be written. 00069 * @param length the length of data to be written from the data parameter. 00070 * @returns the number of bytes written to the buffer, which is 0 if 00071 * the buffer is full. 00072 */ 00073 int write(const char* data, int length); 00074 00075 /** This method attempts to write a single byte to the tx buffer 00076 * within the timeout period. 00077 * 00078 * @param data the byte to be written as a char. 00079 * @timeoutMillis amount of time in milliseconds to complete operation. 00080 * @returns 1 if the byte was written or 0 if the buffer was full. 00081 */ 00082 int write(char data, unsigned int timeoutMillis); 00083 00084 /** This method writes a signle byte as a char to the Tx or write buffer. 00085 * 00086 * @param data the byte to be written as a char. 00087 * @returns 1 if the byte was written or 0 if the buffer was full. 00088 */ 00089 int write(char data); 00090 00091 /** This method is used to get the space available to write bytes to the Tx buffer. 00092 * 00093 * @returns the number of bytes that can be written, 0 if the buffer is full. 00094 */ 00095 int writeable(); 00096 00097 /** This method enables bulk reads from the Rx or read buffer. If more data is 00098 * requested then available it simply returns all remaining data within the 00099 * buffer. 00100 * 00101 * @param data the buffer where data read will be added to. 00102 * @param length the amount of data in bytes to be read into the buffer. 00103 * @timeoutMillis amount of time to complete operation. 00104 * @returns the total number of bytes that were read. 00105 */ 00106 int read(char* data, int length, unsigned int timeoutMillis); 00107 00108 /** This method enables bulk reads from the Rx or read buffer. If more data is 00109 * requested then available it simply returns all remaining data within the 00110 * buffer. 00111 * 00112 * @param data the buffer where data read will be added to. 00113 * @param length the amount of data in bytes to be read into the buffer. 00114 * @returns the total number of bytes that were read. 00115 */ 00116 int read(char* data, int length); 00117 00118 /** This method reads a single byte from the Rx or read buffer. 00119 * 00120 * @param data char where the read byte will be stored. 00121 * @timeoutMillis amount of time to complete operation. 00122 * @returns 1 if byte is read or 0 if no byte is available. 00123 */ 00124 int read(char& data, unsigned int timeoutMillis); 00125 00126 /** This method reads a single byte from the Rx or read buffer. 00127 * 00128 * @param data char where the read byte will be stored. 00129 * @returns 1 if byte is read or 0 if no byte is available. 00130 */ 00131 int read(char& data); 00132 00133 /** This method is used to get the number of bytes available to read from 00134 * the Rx or read buffer. 00135 * 00136 * @returns the number of bytes available, 0 if there are no bytes to read. 00137 */ 00138 int readable(); 00139 00140 /** This method determines if the Tx or write buffer is empty. 00141 * 00142 * @returns true if empty, otherwise false. 00143 */ 00144 bool txEmpty(); 00145 00146 /** This method determines if the Rx or read buffer is empty. 00147 * 00148 * @returns true if empty, otherwise false. 00149 */ 00150 bool rxEmpty(); 00151 00152 /** This method determines if the Tx or write buffer is full. 00153 * 00154 * @returns true if full, otherwise false. 00155 */ 00156 bool txFull(); 00157 00158 /** This method determines if the Rx or read buffer is full. 00159 * 00160 * @returns true if full, otherwise false. 00161 */ 00162 bool rxFull(); 00163 00164 /** This method clears all the data from the internal Tx or write buffer. 00165 */ 00166 virtual void txClear(); 00167 00168 /** This method clears all the data from the internal Rx or read buffer. 00169 */ 00170 virtual void rxClear(); 00171 00172 /** This abstract method should be used by the deriving class to transfer 00173 * data from the internal write buffer (txBuffer) to the physical interface. 00174 * Note that this function is called everytime new data is written to the 00175 * txBuffer though one of the write calls. 00176 */ 00177 virtual void handleWrite() = 0; 00178 00179 /** This abstract method should be used by the deriving class to transfer 00180 * data from the physical interface ot the internal read buffer (rxBuffer). 00181 * Note that this function is never called in this class and typically should 00182 * be called as part of a receive data interrupt routine. 00183 */ 00184 virtual void handleRead() = 0; 00185 00186 protected: 00187 MTSCircularBuffer txBuffer; // Internal write or transmit circular buffer 00188 MTSCircularBuffer rxBuffer; // Internal read or receieve circular buffer 00189 }; 00190 00191 } 00192 00193 #endif /* MTSBUFFEREDIO_H */
Generated on Tue Jul 12 2022 21:46:23 by
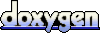