
Read and report XYZ axes from on-board accelerometer for graphing via serial
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "MMA8451Q.h" 00003 00004 // define I2C Pins and address for KL25Z. Taken from default sample code. 00005 PinName const SDA = PTE25; 00006 PinName const SCL = PTE24; 00007 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00008 00009 //serial connection to PC via USB 00010 Serial pc(USBTX, USBRX); 00011 00012 int main(void) 00013 { 00014 //configure on-board I2C accelerometer on KL25Z 00015 MMA8451Q acc(SDA, SCL, MMA8451_I2C_ADDRESS); 00016 //map read acceleration to PWM output on red status LED 00017 PwmOut rled(LED_RED); 00018 float x,y,z; 00019 while (true) { 00020 float x, y, z; 00021 x = acc.getAccX(); 00022 y = acc.getAccY(); 00023 z = acc.getAccZ(); 00024 wait(0.05f); 00025 pc.printf("%1.2f %1.2f %1.2f\n", x, y, z); 00026 } 00027 } 00028 00029 00030 /* *** PROCESSING SKETCH 00031 // Three-axis Graphing sketch 00032 00033 00034 // This program takes ASCII-encoded strings containing floating point numbers 00035 // from the serial port at 9600 baud and graphs them. It expects values in the 00036 // range -1.0 to 1.0, followed by a newline, or newline and carriage return 00037 00038 // Created 20 Apr 2005 00039 // Updated 18 Jan 2008 by Tom Igoe 00040 // Adapted 16 Sep 2014 by Bjoern Hartmann for mbed 00041 // This example code is in the public domain. 00042 00043 import processing.serial.*; 00044 import java.util.Scanner; 00045 00046 Serial myPort; // The serial port 00047 int xPos = 1; // horizontal position of the graph 00048 float lastX =0.f, lastY=0.f, lastZ=0.f; 00049 float minVal=-1.0; 00050 float maxVal=1.0; 00051 00052 void setup () { 00053 // set the window size: 00054 size(400, 300); 00055 00056 // List all the available serial ports 00057 println(Serial.list()); 00058 // Open whatever port is the one you're using. 00059 myPort = new Serial(this, "/dev/tty.usbmodem1412", 9600); 00060 // don't generate a serialEvent() unless you get a newline character: 00061 myPort.bufferUntil('\n'); 00062 // set inital background: 00063 background(200); 00064 } 00065 void draw () { 00066 // everything happens in the serialEvent() 00067 } 00068 00069 void serialEvent (Serial myPort) { 00070 // get the ASCII string: 00071 String inString = myPort.readStringUntil('\n'); 00072 00073 if (inString != null) { 00074 //use the Scanner class to parse 3 floats on a line. 00075 Scanner scanner = new Scanner(inString); 00076 float xAcc=0.0,yAcc=0.0,zAcc=0.0; 00077 try{ 00078 xAcc = scanner.nextFloat(); 00079 yAcc = scanner.nextFloat(); 00080 zAcc = scanner.nextFloat(); 00081 00082 } catch (Exception e) { 00083 print(e); //InputMismatchException 00084 } 00085 00086 //draw x line in red 00087 float xAccScreen = height - map(xAcc, minVal, maxVal, 0, height); 00088 stroke(255,0,0); 00089 line(xPos-1,lastX,xPos,xAccScreen); 00090 00091 //draw y line in green 00092 float yAccScreen = height - map(yAcc, minVal, maxVal, 0, height); 00093 stroke(0,196,0); 00094 line(xPos-1,lastY,xPos,yAccScreen); 00095 00096 //draw z line in blue 00097 float zAccScreen = height - map(zAcc, minVal, maxVal, 0, height); 00098 stroke(0,0,255); 00099 line(xPos-1,lastZ,xPos,zAccScreen); 00100 00101 // at the edge of the screen, go back to the beginning: 00102 if (xPos >= width) { 00103 xPos = 0; 00104 background(200); 00105 } else { 00106 // increment the horizontal position: 00107 xPos++; 00108 } 00109 00110 lastX = xAccScreen; 00111 lastY = yAccScreen; 00112 lastZ = zAccScreen; 00113 00114 } 00115 } 00116 */
Generated on Wed Jul 13 2022 16:54:59 by
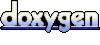