This is the Interface library for WIZnet W5500 chip which forked of EthernetInterfaceW5500, WIZnetInterface and WIZ550ioInterface. This library has simple name as "W5500Interface". and can be used for Wiz550io users also.
Dependents: EvrythngApi Websocket_Ethernet_HelloWorld_W5500 Websocket_Ethernet_W5500 CurrentWeatherData_W5500 ... more
EthernetInterface.h
00001 // EthernetInterface for W5500 2014/8/20 00002 00003 #pragma once 00004 #include "wiznet.h" 00005 00006 /** Interface using Wiznet W5500 chip to connect to an IP-based network 00007 * 00008 */ 00009 00010 00011 class EthernetInterface: public WIZnet_Chip { 00012 public: 00013 00014 /** 00015 * Constructor 00016 * 00017 * \param mosi mbed pin to use for SPI 00018 * \param miso mbed pin to use for SPI 00019 * \param sclk mbed pin to use for SPI 00020 * \param cs chip select of the WIZnet_Chip 00021 * \param reset reset pin of the WIZnet_Chip 00022 */ 00023 EthernetInterface(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset); 00024 EthernetInterface(SPI* spi, PinName cs, PinName reset); 00025 00026 /** Initialize the interface with DHCP w/o MAC address 00027 * Initialize the interface and configure it to use DHCP (no connection at this point). 00028 * \return 0 on success, a negative number on failure 00029 */ 00030 int init(); //With DHCP 00031 /** Initialize the interface with DHCP. 00032 * Initialize the interface and configure it to use DHCP (no connection at this point). 00033 * \param mac the MAC address to use 00034 * \return 0 on success, a negative number on failure 00035 */ 00036 int init(uint8_t * mac); //With DHCP 00037 00038 /** Initialize the interface with a static IP address without MAC. 00039 * Initialize the interface and configure it with the following static configuration (no connection at this point). 00040 * \param ip the IP address to use 00041 * \param mask the IP address mask 00042 * \param gateway the gateway to use 00043 * \return 0 on success, a negative number on failure 00044 */ 00045 00046 int init(const char* ip, const char* mask, const char* gateway); 00047 /** Initialize the interface with a static IP address. 00048 * Initialize the interface and configure it with the following static configuration (no connection at this point). 00049 * \param mac the MAC address to use 00050 * \param ip the IP address to use 00051 * \param mask the IP address mask 00052 * \param gateway the gateway to use 00053 * \return 0 on success, a negative number on failure 00054 */ 00055 int init(uint8_t * mac, const char* ip, const char* mask, const char* gateway); 00056 00057 /** Connect 00058 * Bring the interface up, start DHCP if needed. 00059 * \return 0 on success, a negative number on failure 00060 */ 00061 int connect(); 00062 00063 /** Disconnect 00064 * Bring the interface down 00065 * \return 0 on success, a negative number on failure 00066 */ 00067 int disconnect(); 00068 00069 /** Get IP address 00070 * 00071 * @ returns ip address 00072 */ 00073 char* getIPAddress(); 00074 char* getNetworkMask(); 00075 char* getGateway(); 00076 char* getMACAddress(); 00077 00078 int IPrenew(int timeout_ms = 15*1000); 00079 00080 private: 00081 char ip_string[17]; 00082 char mask_string[17]; 00083 char gw_string[17]; 00084 char mac_string[20]; 00085 bool ip_set; 00086 void getip(void); 00087 }; 00088 00089 #include "TCPSocketConnection.h" 00090 #include "TCPSocketServer.h" 00091 #include "UDPSocket.h"
Generated on Thu Jul 14 2022 02:02:03 by
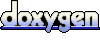