Host library for controlling a WiConnect enabled Wi-Fi module.
Dependents: wiconnect-ota_example wiconnect-web_setup_example wiconnect-test-console wiconnect-tcp_server_example ... more
WiconnectUdpServer.cpp
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 #include <stdarg.h> 00030 00031 #include "Wiconnect.h" 00032 #include "internal/common.h" 00033 #include "api/StringUtil.h" 00034 00035 00036 #define CHECK_CONNECTED() if(!isConnected()) return WICONNECT_NOT_CONNECTED 00037 00038 extern WiconnectResult readerCallback(void *user, void *data, int maxReadSize, int *bytesRead); 00039 00040 00041 /*************************************************************************************************/ 00042 WiconnectUdpServer::WiconnectUdpServer(int rxBufferLen_, void *rxBuffer_, int txBufferLen_, void *txBuffer_) : 00043 WiconnectSocket(rxBufferLen_, rxBuffer_, txBufferLen_, txBuffer_) 00044 { 00045 } 00046 00047 /*************************************************************************************************/ 00048 WiconnectResult WiconnectUdpServer::getRemoteClient(uint32_t *ipAddress, uint16_t *port) 00049 { 00050 WiconnectResult result; 00051 00052 CHECK_CONNECTED(); 00053 CHECK_OTHER_COMMAND_EXECUTING(); 00054 00055 if(WICONNECT_SUCCEEDED(result, wiconnect->sendCommand("udps read -q"))) 00056 { 00057 char *portStr = strchr(wiconnect->internalBuffer, ','); 00058 if(portStr == NULL) 00059 { 00060 result = WICONNECT_RESPONSE_PARSE_ERROR; 00061 } 00062 else 00063 { 00064 *portStr++ = 0; 00065 Wiconnect::strToIp(wiconnect->internalBuffer, ipAddress); 00066 StringUtil::strToUint16(portStr, port); 00067 } 00068 } 00069 00070 CHECK_CLEANUP_COMMAND(); 00071 00072 return result; 00073 } 00074 00075 /*************************************************************************************************/ 00076 WiconnectResult WiconnectUdpServer::setRemoteClient(const char* _host, uint16_t port) 00077 { 00078 WiconnectResult result; 00079 uint32_t ip; 00080 00081 if(WICONNECT_SUCCEEDED(result, wiconnect->lookup(_host, &ip))) 00082 { 00083 ip = htonl(ip); // due to a bug in the firmware, need to reserve byte order 00084 Wiconnect::ipToStr(ip, host); 00085 remotePort = port; 00086 } 00087 00088 return result; 00089 } 00090 00091 /*************************************************************************************************/ 00092 WiconnectResult WiconnectUdpServer::setRemoteClient(uint32_t ip, uint16_t port) 00093 { 00094 ip = htonl(ip); // due to a bug in the firmware, need to reserve byte order 00095 Wiconnect::ipToStr(ip, host); 00096 remotePort = port; 00097 return WICONNECT_SUCCESS; 00098 } 00099 00100 /*************************************************************************************************/ 00101 WiconnectResult WiconnectUdpServer::flushTxBuffer() 00102 { 00103 WiconnectResult result = WICONNECT_SUCCESS; 00104 00105 CHECK_CONNECTED(); 00106 00107 if(txBuffer.size == 0) 00108 { 00109 CHECK_OTHER_COMMAND_EXECUTING(); 00110 } 00111 00112 00113 if(txBuffer.bytesPending > 0) 00114 { 00115 loop: 00116 if(host[0] != 0) 00117 { 00118 result = wiconnect->sendCommand(ReaderFunc(readerCallback), &this->txBuffer, "udps write %u %s %u", txBuffer.bytesPending, host, remotePort); 00119 } 00120 else 00121 { 00122 result = wiconnect->sendCommand(ReaderFunc(readerCallback), &this->txBuffer, "udps write %u", txBuffer.bytesPending); 00123 } 00124 00125 // if still processing and in non-blocking mode and using a txtBuffer, 00126 // then this api call must block until all the data is sent 00127 if(result == WICONNECT_PROCESSING && wiconnect->nonBlocking && txBuffer.size > 0) 00128 { 00129 goto loop; 00130 } 00131 } 00132 00133 if(txBuffer.size == 0) 00134 { 00135 CHECK_CLEANUP_COMMAND(); 00136 } 00137 00138 if(result != WICONNECT_PROCESSING) 00139 { 00140 txBuffer.ptr = txBuffer.buffer; 00141 txBuffer.bytesPending = 0; 00142 } 00143 00144 return result; 00145 }
Generated on Tue Jul 12 2022 17:35:59 by
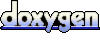