Host library for controlling a WiConnect enabled Wi-Fi module.
Dependents: wiconnect-ota_example wiconnect-web_setup_example wiconnect-test-console wiconnect-tcp_server_example ... more
WiconnectCommand.cpp
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 00030 #include "internal/CommandCommon.h" 00031 #include "api/StringUtil.h" 00032 00033 00034 00035 /*************************************************************************************************/ 00036 void Wiconnect::setCommandDefaultTimeout(TimerTimeout timeoutMs) 00037 { 00038 defaultTimeoutMs = timeoutMs; 00039 } 00040 00041 /*************************************************************************************************/ 00042 TimerTimeout Wiconnect::getCommandDefaultTimeout() 00043 { 00044 return defaultTimeoutMs; 00045 } 00046 00047 /*************************************************************************************************/ 00048 void Wiconnect::setBlockingEnabled(bool blockingEnabled) 00049 { 00050 nonBlocking = !blockingEnabled; 00051 } 00052 00053 /*************************************************************************************************/ 00054 bool Wiconnect::getBlockingEnabled(void) 00055 { 00056 return !nonBlocking; 00057 } 00058 00059 /*************************************************************************************************/ 00060 char* Wiconnect::getResponseBuffer() 00061 { 00062 return internalBuffer; 00063 } 00064 00065 /*************************************************************************************************/ 00066 uint16_t Wiconnect::getLastCommandResponseLength() 00067 { 00068 CommandHeader *header = (CommandHeader*)commandHeaderBuffer; 00069 return header->response_len; 00070 } 00071 00072 /*************************************************************************************************/ 00073 const char* Wiconnect::getLastCommandResponseCodeStr() 00074 { 00075 if(!initialized) 00076 { 00077 return NULL; 00078 } 00079 static const char* const response_error_strings[] ={ 00080 "Null", 00081 "Success", 00082 "Failed", 00083 "Parse error", 00084 "Unknown command", 00085 "Too few arguments", 00086 "Too many arguments", 00087 "Unknown command option", 00088 "Bad command arguments" 00089 }; 00090 CommandHeader *header = (CommandHeader*)commandHeaderBuffer; 00091 00092 return response_error_strings[header->response_code]; 00093 } 00094 00095 00096 /*************************************************************************************************/ 00097 WiconnectResult Wiconnect::responseToUint32(uint32_t *uint32Ptr) 00098 { 00099 CHECK_INITIALIZED(); 00100 return StringUtil::strToUint32(internalBuffer, uint32Ptr) ? WICONNECT_SUCCESS : WICONNECT_RESPONSE_PARSE_ERROR; 00101 } 00102 00103 00104 /*************************************************************************************************/ 00105 WiconnectResult Wiconnect::responseToInt32(int32_t *int32Ptr) 00106 { 00107 CHECK_INITIALIZED(); 00108 return StringUtil::strToInt32(internalBuffer, int32Ptr) ? WICONNECT_SUCCESS : WICONNECT_RESPONSE_PARSE_ERROR; 00109 } 00110 00111
Generated on Tue Jul 12 2022 17:35:58 by
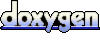