Host library for controlling a WiConnect enabled Wi-Fi module.
Dependents: wiconnect-ota_example wiconnect-web_setup_example wiconnect-test-console wiconnect-tcp_server_example ... more
GhmMessageProcessing.cpp
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 00030 #include "Wiconnect.h" 00031 #include "internal/common.h" 00032 #include "api/StringUtil.h" 00033 00034 00035 #define GHM_HOST_URL "api.gohack.me" 00036 00037 00038 /*************************************************************************************************/ 00039 WiconnectResult GhmInterface::ghmPostMessage(WiconnectSocket &socket, bool jsonFormatted) 00040 { 00041 WiconnectResult result; 00042 int32_t handle; 00043 char *cmdBuffer = wiconnect->internalBuffer; 00044 00045 if(WICONNECT_IS_IDLE()) 00046 { 00047 strcpy(cmdBuffer, "gme post "); 00048 if(jsonFormatted) 00049 { 00050 strcat(cmdBuffer, "json"); 00051 } 00052 } 00053 00054 CHECK_OTHER_COMMAND_EXECUTING(); 00055 00056 if(WICONNECT_SUCCEEDED(result, wiconnect->sendCommand(cmdBuffer))) 00057 { 00058 if(!WICONNECT_FAILED(result, wiconnect->responseToInt32(&handle))) 00059 { 00060 socket.init(handle, SOCKET_TYPE_GHM, GHM_HOST_URL, 443, SOCKET_ANY_PORT); 00061 } 00062 } 00063 00064 CHECK_CLEANUP_COMMAND(); 00065 00066 return result; 00067 } 00068 00069 /*************************************************************************************************/ 00070 WiconnectResult GhmInterface::ghmGetMessage(WiconnectSocket &socket, GhmMessageGetType getType) 00071 { 00072 return ghmGetMessage(socket, 255, NULL, getType); 00073 } 00074 00075 /*************************************************************************************************/ 00076 WiconnectResult GhmInterface::ghmGetMessage(WiconnectSocket &socket, uint8_t listIndex, GhmMessageGetType getType) 00077 { 00078 return ghmGetMessage(socket, listIndex, NULL, getType); 00079 } 00080 00081 /*************************************************************************************************/ 00082 WiconnectResult GhmInterface::ghmGetMessage(WiconnectSocket &socket, const char *msgId, GhmMessageGetType getType) 00083 { 00084 return ghmGetMessage(socket, 255, msgId, getType); 00085 } 00086 00087 /*************************************************************************************************/ 00088 WiconnectResult GhmInterface::ghmGetMessage(WiconnectSocket &socket, uint8_t listIndex, const char *msgId, GhmMessageGetType getType) 00089 { 00090 WiconnectResult result; 00091 int32_t handle; 00092 char *cmdBuffer = wiconnect->internalBuffer; 00093 00094 if(WICONNECT_IS_IDLE()) 00095 { 00096 char *ptr = &cmdBuffer[7]; 00097 strcpy(cmdBuffer, "gme get"); 00098 if(listIndex != 255) 00099 { 00100 sprintf(ptr, " %u", listIndex); 00101 } 00102 else if(msgId != NULL) 00103 { 00104 sprintf(ptr, " %s", msgId); 00105 } 00106 00107 if(getType == GHM_MSG_GET_BODY) 00108 { 00109 strcat(cmdBuffer, " body"); 00110 } 00111 else if(getType == GHM_MSG_GET_ALL) 00112 { 00113 strcat(cmdBuffer, " all"); 00114 } 00115 } 00116 00117 CHECK_OTHER_COMMAND_EXECUTING(); 00118 00119 if(WICONNECT_SUCCEEDED(result, wiconnect->sendCommand(cmdBuffer))) 00120 { 00121 if(!WICONNECT_FAILED(result, wiconnect->responseToInt32(&handle))) 00122 { 00123 socket.init(handle, SOCKET_TYPE_GHM, GHM_HOST_URL, 443, SOCKET_ANY_PORT); 00124 } 00125 } 00126 00127 CHECK_CLEANUP_COMMAND(); 00128 00129 return result; 00130 } 00131 00132 /*************************************************************************************************/ 00133 WiconnectResult GhmInterface::ghmDeleteMessage(uint8_t listIndex) 00134 { 00135 WiconnectResult result; 00136 char *cmdBuffer = wiconnect->internalBuffer; 00137 00138 if(WICONNECT_IS_IDLE()) 00139 { 00140 sprintf(cmdBuffer, "gme delete %u", listIndex); 00141 } 00142 00143 CHECK_OTHER_COMMAND_EXECUTING(); 00144 00145 result = wiconnect->sendCommand(cmdBuffer); 00146 00147 CHECK_CLEANUP_COMMAND(); 00148 00149 return result; 00150 } 00151 00152 /*************************************************************************************************/ 00153 WiconnectResult GhmInterface::ghmDeleteMessage(const char *msgId) 00154 { 00155 WiconnectResult result; 00156 char *cmdBuffer = wiconnect->internalBuffer; 00157 00158 if(WICONNECT_IS_IDLE()) 00159 { 00160 sprintf(cmdBuffer, "gme delete %s", msgId); 00161 } 00162 00163 CHECK_OTHER_COMMAND_EXECUTING(); 00164 00165 result = wiconnect->sendCommand(cmdBuffer); 00166 00167 CHECK_CLEANUP_COMMAND(); 00168 00169 return result; 00170 } 00171 00172 /*************************************************************************************************/ 00173 WiconnectResult GhmInterface::ghmListMessages(GhmMessageList &msgList, uint8_t maxCount) 00174 { 00175 WiconnectResult result; 00176 char *cmdBuffer = wiconnect->internalBuffer; 00177 00178 if(WICONNECT_IS_IDLE()) 00179 { 00180 msgList.reset(); 00181 strcpy(cmdBuffer, "gme list"); 00182 if(maxCount != 0) 00183 { 00184 sprintf(&cmdBuffer[8], " -c %u", maxCount); 00185 } 00186 } 00187 00188 CHECK_OTHER_COMMAND_EXECUTING(); 00189 00190 if(WICONNECT_SUCCEEDED(result, wiconnect->sendCommand(cmdBuffer))) 00191 { 00192 result = processMessageList(wiconnect->internalBuffer, msgList); 00193 } 00194 00195 CHECK_CLEANUP_COMMAND(); 00196 00197 return result; 00198 } 00199 00200 00201 /*************************************************************************************************/ 00202 WiconnectResult GhmInterface::processMessageList(char *resultStr, GhmMessageList &resultList) 00203 { 00204 WiconnectResult result = WICONNECT_SUCCESS; 00205 char *line, *savedLine; 00206 00207 for(savedLine = resultStr; (line = StringUtil::strtok_r(savedLine, "\r\n", &savedLine)) != NULL;) 00208 { 00209 char *toks[4], *savedTok; 00210 00211 if(*line != '#') 00212 { 00213 continue; 00214 } 00215 savedTok = line + 2; 00216 00217 for(int i = 0; i < 4 && (toks[i] = StringUtil::strtok_r(savedTok, " ", &savedTok)) != NULL; ++i) 00218 { 00219 if(toks[i] == NULL) 00220 { 00221 result = WICONNECT_RESPONSE_PARSE_ERROR; 00222 goto exit; 00223 } 00224 } 00225 00226 if(WICONNECT_FAILED(result, resultList.add(toks[1], toks[2], toks[3]))) 00227 { 00228 goto exit; 00229 } 00230 } 00231 00232 exit: 00233 return result; 00234 }
Generated on Tue Jul 12 2022 17:35:58 by
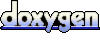