Host library for controlling a WiConnect enabled Wi-Fi module.
Dependents: wiconnect-ota_example wiconnect-web_setup_example wiconnect-test-console wiconnect-tcp_server_example ... more
GhmInterface.cpp
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 00030 #include "Wiconnect.h" 00031 #include "internal/common.h" 00032 #include "api/StringUtil.h" 00033 00034 00035 /*************************************************************************************************/ 00036 GhmInterface::GhmInterface(Wiconnect *wiconnect_) 00037 { 00038 wiconnect = wiconnect_; 00039 } 00040 00041 /*************************************************************************************************/ 00042 WiconnectResult GhmInterface::ghmDownloadCapabilities(const char *capsNameOrCustomUrl, uint32_t version) 00043 { 00044 WiconnectResult result; 00045 char *cmdBuffer = wiconnect->internalBuffer; 00046 00047 if(WICONNECT_IS_IDLE()) 00048 { 00049 strcpy(cmdBuffer, "gca download "); 00050 if(capsNameOrCustomUrl != NULL) 00051 { 00052 strcat(cmdBuffer, capsNameOrCustomUrl); 00053 if(version > 0) 00054 { 00055 char temp[16]; 00056 char *ptr = cmdBuffer + strlen(cmdBuffer); 00057 *ptr++ = ' '; 00058 sprintf(ptr, "%s", Wiconnect::fileVersionIntToStr(version, true, temp)); 00059 } 00060 } 00061 } 00062 00063 CHECK_OTHER_COMMAND_EXECUTING(); 00064 00065 result = wiconnect->sendCommand(cmdBuffer); 00066 00067 CHECK_CLEANUP_COMMAND(); 00068 00069 return result; 00070 } 00071 00072 /*************************************************************************************************/ 00073 WiconnectResult GhmInterface::ghmActivate(const char *userName, const char *password, const char *capsFilename) 00074 { 00075 WiconnectResult result; 00076 char *cmdBuffer = wiconnect->internalBuffer; 00077 00078 if(WICONNECT_IS_IDLE()) 00079 { 00080 sprintf(cmdBuffer, "gac %s %s %s", userName, password, (capsFilename == NULL) ? "" : capsFilename); 00081 } 00082 00083 CHECK_OTHER_COMMAND_EXECUTING(); 00084 00085 result = wiconnect->sendCommand(cmdBuffer); 00086 00087 CHECK_CLEANUP_COMMAND(); 00088 00089 return result; 00090 } 00091 00092 /*************************************************************************************************/ 00093 WiconnectResult GhmInterface::ghmDeactivate(const char *userName, const char *password) 00094 { 00095 WiconnectResult result; 00096 char *cmdBuffer = wiconnect->internalBuffer; 00097 00098 if(WICONNECT_IS_IDLE()) 00099 { 00100 sprintf(cmdBuffer, "gac %s %s", userName, password); 00101 } 00102 00103 CHECK_OTHER_COMMAND_EXECUTING(); 00104 00105 result = wiconnect->sendCommand(cmdBuffer); 00106 00107 CHECK_CLEANUP_COMMAND(); 00108 00109 return result; 00110 } 00111 00112 /*************************************************************************************************/ 00113 WiconnectResult GhmInterface::ghmIsActivated(bool *statusPtr) 00114 { 00115 WiconnectResult result; 00116 uint32_t status; 00117 00118 if(WICONNECT_SUCCEEDED(result, wiconnect->getSetting("ghm.status", &status))) 00119 { 00120 *statusPtr = (bool)status; 00121 } 00122 00123 return result; 00124 } 00125 00126 00127 /*************************************************************************************************/ 00128 WiconnectResult GhmInterface::ghmRead(const char *controlName, const char **valueStrPtr) 00129 { 00130 WiconnectResult result; 00131 char *cmdBuffer = wiconnect->internalBuffer; 00132 00133 if(WICONNECT_IS_IDLE()) 00134 { 00135 sprintf(cmdBuffer, "gre %s", controlName); 00136 } 00137 00138 CHECK_OTHER_COMMAND_EXECUTING(); 00139 00140 if(WICONNECT_SUCCEEDED(result, wiconnect->sendCommand(cmdBuffer))) 00141 { 00142 *valueStrPtr = wiconnect->internalBuffer; 00143 } 00144 00145 CHECK_CLEANUP_COMMAND(); 00146 00147 return result; 00148 } 00149 00150 /*************************************************************************************************/ 00151 WiconnectResult GhmInterface::ghmRead(const char *controlName, uint32_t *valueIntPtr) 00152 { 00153 WiconnectResult result; 00154 char *cmdBuffer = wiconnect->internalBuffer; 00155 00156 if(WICONNECT_IS_IDLE()) 00157 { 00158 sprintf(cmdBuffer, "gre %s", controlName); 00159 } 00160 00161 CHECK_OTHER_COMMAND_EXECUTING(); 00162 00163 if(WICONNECT_SUCCEEDED(result, wiconnect->sendCommand(cmdBuffer))) 00164 { 00165 result = wiconnect->responseToUint32(valueIntPtr); 00166 } 00167 00168 CHECK_CLEANUP_COMMAND(); 00169 00170 return result; 00171 } 00172 00173 /*************************************************************************************************/ 00174 WiconnectResult GhmInterface::ghmRead(const char *controlName, char *valueBuffer, uint16_t valueBufferLen) 00175 { 00176 WiconnectResult result; 00177 char *cmdBuffer = wiconnect->internalBuffer; 00178 00179 if(WICONNECT_IS_IDLE()) 00180 { 00181 sprintf(cmdBuffer, "gre %s", controlName); 00182 } 00183 00184 CHECK_OTHER_COMMAND_EXECUTING(); 00185 00186 result = wiconnect->sendCommand(valueBuffer, valueBufferLen, cmdBuffer); 00187 00188 CHECK_CLEANUP_COMMAND(); 00189 00190 return result; 00191 } 00192 00193 /*************************************************************************************************/ 00194 WiconnectResult GhmInterface::ghmWrite(const char *elementName, const char *strValue) 00195 { 00196 WiconnectResult result; 00197 char *cmdBuffer = wiconnect->internalBuffer; 00198 00199 if(WICONNECT_IS_IDLE()) 00200 { 00201 sprintf(cmdBuffer, "gwr %s %s", elementName, strValue); 00202 } 00203 00204 CHECK_OTHER_COMMAND_EXECUTING(); 00205 00206 result = wiconnect->sendCommand(cmdBuffer); 00207 00208 CHECK_CLEANUP_COMMAND(); 00209 00210 return result; 00211 } 00212 00213 /*************************************************************************************************/ 00214 WiconnectResult GhmInterface::ghmWrite(const char *elementName, uint32_t uintValue) 00215 { 00216 WiconnectResult result; 00217 char *cmdBuffer = wiconnect->internalBuffer; 00218 00219 if(WICONNECT_IS_IDLE()) 00220 { 00221 sprintf(cmdBuffer, "gwr %s %lu", elementName, uintValue); 00222 } 00223 00224 CHECK_OTHER_COMMAND_EXECUTING(); 00225 00226 result = wiconnect->sendCommand(cmdBuffer); 00227 00228 CHECK_CLEANUP_COMMAND(); 00229 00230 return result; 00231 } 00232 00233 /*************************************************************************************************/ 00234 WiconnectResult GhmInterface::ghmWrite(const char *elementName, int32_t intValue) 00235 { 00236 WiconnectResult result; 00237 char *cmdBuffer = wiconnect->internalBuffer; 00238 00239 if(WICONNECT_IS_IDLE()) 00240 { 00241 sprintf(cmdBuffer, "gwr %s %ld", elementName, intValue); 00242 } 00243 00244 CHECK_OTHER_COMMAND_EXECUTING(); 00245 00246 result = wiconnect->sendCommand(cmdBuffer); 00247 00248 CHECK_CLEANUP_COMMAND(); 00249 00250 return result; 00251 } 00252 00253 /*************************************************************************************************/ 00254 WiconnectResult GhmInterface::ghmWrite(const GhmElementArray *elementArray) 00255 { 00256 WiconnectResult result; 00257 char *cmdBuffer = wiconnect->internalBuffer; 00258 00259 if(WICONNECT_IS_IDLE()) 00260 { 00261 char *ptr = &cmdBuffer[4]; 00262 sprintf(cmdBuffer, "gwr "); 00263 00264 const GhmElement *elEnd = &elementArray->elements[elementArray->count]; 00265 for(const GhmElement *el = &elementArray->elements[0]; el < elEnd; ++el) 00266 { 00267 if(el-> type == GHM_VALUE_INT) 00268 { 00269 ptr += sprintf(ptr, "%s,%lu|", el->elementName, el->u.intValue); 00270 } 00271 else 00272 { 00273 ptr += sprintf(ptr, "%s,%s|", el->elementName, el->u.strValue); 00274 } 00275 } 00276 *(ptr-1) = 0; 00277 } 00278 00279 CHECK_OTHER_COMMAND_EXECUTING(); 00280 00281 result = wiconnect->sendCommand(cmdBuffer); 00282 00283 CHECK_CLEANUP_COMMAND(); 00284 00285 return result; 00286 } 00287 00288 /*************************************************************************************************/ 00289 WiconnectResult GhmInterface::ghmSynchronize(GhmSyncType type) 00290 { 00291 WiconnectResult result; 00292 char *cmdBuffer = wiconnect->internalBuffer; 00293 00294 if(WICONNECT_IS_IDLE()) 00295 { 00296 sprintf(cmdBuffer, "gsy %s", (type == GHM_SYNC_PUSH_ONLY) ? "push" : 00297 (type == GHM_SYNC_PULL_ONLY) ? "pull" : ""); 00298 } 00299 00300 CHECK_OTHER_COMMAND_EXECUTING(); 00301 00302 result = wiconnect->sendCommand(cmdBuffer); 00303 00304 CHECK_CLEANUP_COMMAND(); 00305 00306 return result; 00307 }
Generated on Tue Jul 12 2022 17:35:58 by
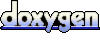