Host library for controlling a WiConnect enabled Wi-Fi module.
Dependents: wiconnect-ota_example wiconnect-web_setup_example wiconnect-test-console wiconnect-tcp_server_example ... more
FileList.cpp
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 00030 00031 #include "Wiconnect.h" 00032 #include "api/types/FileList.h" 00033 00034 00035 00036 /*************************************************************************************************/ 00037 FileList::FileList(int bufferLen_, void *buffer_) 00038 { 00039 count = 0; 00040 listHead = listTail = NULL; 00041 buffer = (uint8_t*)buffer_; 00042 bufferPtr = buffer; 00043 bufferLen = bufferLen_; 00044 } 00045 00046 /*************************************************************************************************/ 00047 FileList::~FileList() 00048 { 00049 if(buffer == NULL) 00050 { 00051 WiconnectFile* result = listHead; 00052 while(result != NULL) 00053 { 00054 WiconnectFile* tmp = result; 00055 result = result->next; 00056 delete tmp; 00057 } 00058 } 00059 } 00060 00061 /*************************************************************************************************/ 00062 WiconnectResult FileList::add(const char *typeStr, const char *flagsStr, const char* sizeStr, const char *versionStr, const char *nameStr) 00063 { 00064 WiconnectResult result; 00065 WiconnectFile *res; 00066 00067 if(buffer == NULL) 00068 { 00069 #ifdef WICONNECT_ENABLE_MALLOC 00070 res = new WiconnectFile(); 00071 if(res == NULL) 00072 #endif 00073 { 00074 return WICONNECT_NULL_BUFFER; 00075 } 00076 } 00077 else 00078 { 00079 if(bufferLen < sizeof(WiconnectFile)) 00080 { 00081 return WICONNECT_OVERFLOW; 00082 } 00083 res = (WiconnectFile*)bufferPtr; 00084 memset(res, 0, sizeof(WiconnectFile)); 00085 bufferLen -= sizeof(WiconnectFile); 00086 bufferPtr += sizeof(WiconnectFile); 00087 } 00088 00089 if(WICONNECT_FAILED(result, res->initWithListing(typeStr, flagsStr, sizeStr, versionStr, nameStr))) 00090 { 00091 if(buffer == NULL) 00092 { 00093 delete res; 00094 } 00095 } 00096 else 00097 { 00098 if(listHead == NULL) 00099 { 00100 listHead = listTail = res; 00101 } 00102 else 00103 { 00104 res->previous = listTail; 00105 listTail->next = res; 00106 listTail = res; 00107 } 00108 ++count; 00109 } 00110 00111 return result; 00112 } 00113 00114 /*************************************************************************************************/ 00115 const WiconnectFile* FileList::getListHead() const 00116 { 00117 return listHead; 00118 } 00119 00120 /*************************************************************************************************/ 00121 int FileList::getCount() const 00122 { 00123 return count; 00124 } 00125 00126 /*************************************************************************************************/ 00127 const WiconnectFile* FileList::getResult(int i) const 00128 { 00129 if(i >= count) 00130 return NULL; 00131 00132 WiconnectFile* result = listHead; 00133 while(i-- != 0) 00134 result = result->next; 00135 00136 return result; 00137 } 00138 00139 /*************************************************************************************************/ 00140 const WiconnectFile* FileList::operator [](int i) const 00141 { 00142 return getResult(i); 00143 } 00144
Generated on Tue Jul 12 2022 17:35:58 by
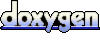